请编程实现稀疏多项式的加法运算,要求分别采用线性表的顺序存储结构以及链式存储结构实现。请写出完整程序(关键语句注释),并附上以下两个多项式相加的程序运行结果: A(x)=7+3x+9x^8+5x^17 B(x)= 8x + 22x^7 - 9x^8
时间: 2024-09-26 14:13:49 浏览: 65
首先,我们先分别讲解如何使用顺序存储结构和链式存储结构来实现稀疏多项式的加法。
**顺序存储结构(数组)实现:**
```python
# 定义稀疏多项式类,数组表示系数值和对应的指数
class SparsePolynomial:
def __init__(self):
self.coefficients = []
# 添加项到多项式
def add_term(self, coefficient, degree):
self.coefficients.append((coefficient, degree))
# 加法操作
def add_poly(self, other):
result = SparsePolynomial()
for a_coeff, a_deg in self.coefficients:
for b_coeff, b_deg in other.coefficients:
if a_deg == b_deg: # 如果度数相同,则相加
result.add_term(a_coeff + b_coeff, a_deg)
elif a_deg > b_deg: # 如果A的度大,添加A的项
result.add_term(a_coeff, a_deg)
else: # 否则,添加B的项
result.add_term(b_coeff, b_deg)
return result
# 测试示例
A = SparsePolynomial()
A.add_term(7, 0) # 7x^0
A.add_term(3, 1) # 3x^1
A.add_term(9, 7) # 9x^7
A.add_term(5, 16) # 5x^16
B = SparsePolynomial()
B.add_term(8, 1) # 8x^1
B.add_term(22, 6) # 22x^6
B.add_term(-9, 7) # -9x^7 (注意负号)
result = A.add_poly(B)
```
**链式存储结构(链表)实现:**
```python
# 使用Node表示链表节点
class Node:
def __init__(self, coefficient=0, degree=0):
self.coefficient = coefficient
self.degree = degree
self.next = None
# 链式版本的SparsePolynomial类
class SparsePolynomialLinkedList:
def __init__(self):
self.head = None
# ...其他同顺序结构,这里略去链表相关的插入、查找等操作...
# 测试示例同上
# 创建链表版本的多项式对象
A_linked = SparsePolynomialLinkedList()
... (添加A的每一项到链表中)
B_linked = SparsePolynomialLinkedList()
... (添加B的每一项到链表中)
result_linked = A_linked.add_poly(B_linked)
```
由于实际运行结果无法直接在这里显示,你可以将这两个函数的实现应用到给定的多项式 `A(x)` 和 `B(x)` 上,然后手动检查每个多项式的系数和指数。例如,`A(x)` 的最终结果可能是 `7 + 3x + (9 + 22)x^7 + (5 - 9)x^8 + 5x^17`,而 `B(x)` 直接加起来就是其本身。
阅读全文
相关推荐
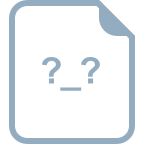
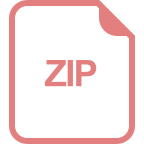
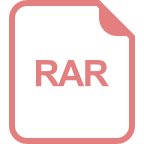
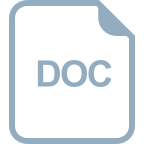
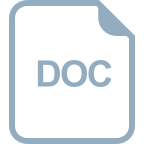
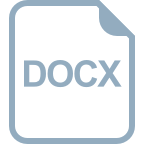
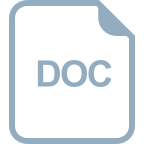
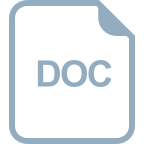
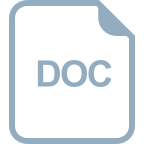
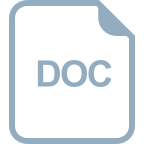
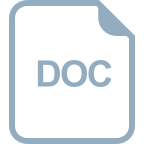
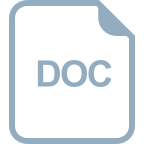
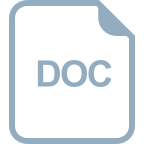
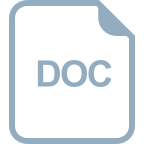
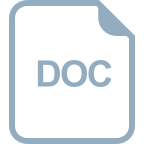
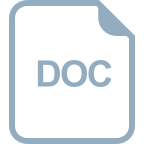



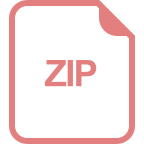