基于stm32f103c8t6写一个驱动超声波的代码
时间: 2023-08-09 11:09:03 浏览: 83
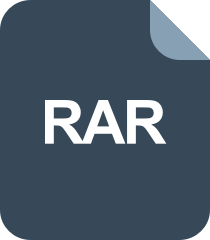
基于STM32的超声波程序
当然可以!以下是一个基于STM32F103C8T6微控制器的超声波驱动代码示例:
```c
#include "stm32f10x.h"
#define TRIG_PIN GPIO_Pin_0
#define ECHO_PIN GPIO_Pin_1
#define TRIG_PORT GPIOA
#define ECHO_PORT GPIOA
void delay_us(uint32_t us) {
us *= (SystemCoreClock / 1000000) / 9;
while (us--) {
asm volatile("nop");
}
}
void delay_ms(uint32_t ms) {
ms *= (SystemCoreClock / 1000) / 9;
while (ms--) {
asm volatile("nop");
}
}
void init_timer() {
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM2, ENABLE);
TIM_TimeBaseInitTypeDef timerInitStructure;
timerInitStructure.TIM_Prescaler = ((SystemCoreClock / 1000000) / 2) - 1;
timerInitStructure.TIM_CounterMode = TIM_CounterMode_Up;
timerInitStructure.TIM_Period = 0xFFFF;
timerInitStructure.TIM_ClockDivision = TIM_CKD_DIV1;
TIM_TimeBaseInit(TIM2, &timerInitStructure);
TIM_Cmd(TIM2, ENABLE);
}
void init_gpio() {
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitTypeDef gpioInitStructure;
gpioInitStructure.GPIO_Pin = TRIG_PIN;
gpioInitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
gpioInitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(TRIG_PORT, &gpioInitStructure);
gpioInitStructure.GPIO_Pin = ECHO_PIN;
gpioInitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(ECHO_PORT, &gpioInitStructure);
}
float measure_distance() {
GPIO_SetBits(TRIG_PORT, TRIG_PIN);
delay_us(10);
GPIO_ResetBits(TRIG_PORT, TRIG_PIN);
while (!GPIO_ReadInputDataBit(ECHO_PORT, ECHO_PIN));
TIM_SetCounter(TIM2, 0);
while (GPIO_ReadInputDataBit(ECHO_PORT, ECHO_PIN));
uint16_t pulse_width = TIM_GetCounter(TIM2);
float distance = pulse_width * 0.0343 / 2;
return distance;
}
int main(void) {
SystemInit();
init_gpio();
init_timer();
while (1) {
float distance = measure_distance();
// 在此处可以使用测量到的距离进行其他操作
// 例如,将其通过串口发送给PC或其他设备
delay_ms(500);
}
}
```
这段代码初始化了超声波模块的引脚,使用定时器2来测量脉冲宽度,并计算距离。在主循环中,它不断测量距离并执行其他操作(例如通过串口发送数据),然后延迟500毫秒。
请注意,此代码仅为示例,您可能需要根据您的具体硬件和需求进行适当的修改和调整。
阅读全文
相关推荐
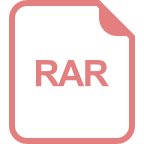
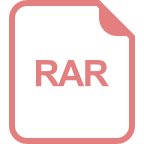
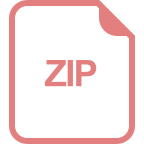
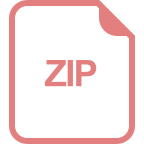






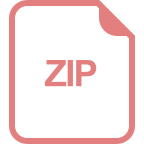
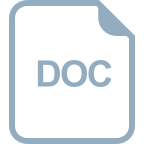


