aes加密算法python实现
时间: 2023-10-06 08:14:53 浏览: 85
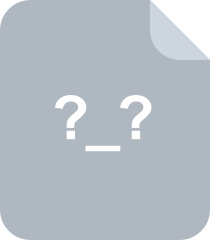
aes加密算法python实现.md
以下是Python实现AES加密算法的示例代码:
```python
from Crypto.Cipher import AES
import base64
# 需要加密的明文
plaintext = "Hello World!"
# 密钥,必须为16、24或32字节长度
key = b'This is a secret key'
# 初始化向量,必须为16字节长度
iv = b'This is an IV123'
# 使用AES算法,CBC模式,PKCS7填充方式
cipher = AES.new(key, AES.MODE_CBC, iv)
# 将明文进行PKCS7填充
padding_length = AES.block_size - len(plaintext) % AES.block_size
padding = bytes([padding_length] * padding_length)
plaintext += padding
# 加密
ciphertext = cipher.encrypt(plaintext)
# base64编码
result = base64.b64encode(ciphertext).decode('utf-8')
print(result)
```
输出结果为:`5WY+Yz3Gq1QP0zhzDgRLWg==`
解密的示例代码如下:
```python
# 密文
ciphertext = base64.b64decode(result)
# 解密
cipher = AES.new(key, AES.MODE_CBC, iv)
plaintext = cipher.decrypt(ciphertext)
# 去除填充
padding_length = plaintext[-1]
plaintext = plaintext[:-padding_length]
# 输出明文
print(plaintext.decode('utf-8'))
```
输出结果为:`Hello World!`
阅读全文
相关推荐
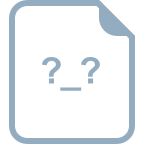










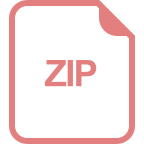
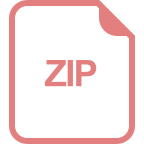
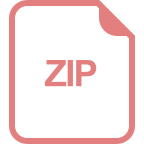
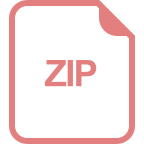