用c++实现单链表的头插法建表、尾插法建表、输出数据元素、按位查找、按值查找、插入数据元素、删除数据元素的操作
时间: 2024-09-22 18:10:28 浏览: 48
在 C++ 中,我们可以使用结构体和指针来实现单链表的基本操作:
1. **头插法建表 (Insert at Head)**:
```cpp
struct Node {
int data;
Node* next;
};
// 插入新节点到头部
void insertAtHead(Node** head, int value) {
Node* newNode = new Node();
newNode->data = value;
newNode->next = *head; // 将新节点的 next 指向当前头节点
*head = newNode; // 更新头节点指向新节点
}
```
2. **尾插法建表 (Insert at Tail)**:
```cpp
// 在尾部插入新节点
void insertAtTail(Node** head, int value) {
if (*head == nullptr) {
insertAtHead(head, value); // 如果链表为空,直接插入头
} else {
Node* current = *head;
while (current->next != nullptr) {
current = current->next;
}
current->next = new Node(); // 新节点的 next 指向 nullptr
current->next->data = value;
}
}
```
3. **输出数据元素 (Traversal)**:
```cpp
void printList(Node* head) {
while (head != nullptr) {
cout << head->data << " ";
head = head->next;
}
cout << endl;
}
```
4. **按位查找 (Binary Search - 链表不适合,因为链表通常无序)**: 二分查找适用于有序数组,对链表不适用。
5. **按值查找 (Search by Value)**:
```cpp
Node* searchByValue(Node* head, int value) {
while (head != nullptr && head->data != value) {
head = head->next;
}
return head;
}
```
6. **插入数据元素 (Insert Data)**: 已经分别有头插法和尾插法的示例。
7. **删除数据元素 (Delete Data)**:
```cpp
void deleteNode(Node** head, int value) {
if (*head == nullptr) return;
if (*head->data == value) { // 删除头节点
Node* temp = *head;
*head = *head->next;
delete temp;
return;
}
Node* current = *head;
while (current->next != nullptr && current->next->data != value) {
current = current->next;
}
if (current->next != nullptr) {
Node* toBeDeleted = current->next;
current->next = current->next->next;
delete toBeDeleted;
}
}
```
相关推荐
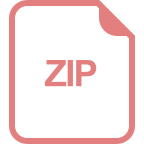
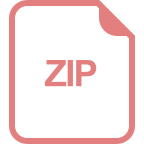
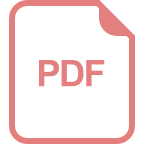














