c++如何判断一个整数是不是它的质因数的整数次幂,这个质因数但不包括它本身
时间: 2024-09-28 10:09:36 浏览: 31
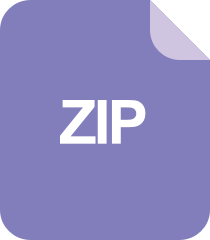
蓝桥杯c++-蓝桥杯竞赛练习之基础练习题分解质因数.zip
在 C++ 中,判断一个整数是否为某个质因数的整数次幂通常涉及分解质因数和循环检测。你可以通过以下步骤来实现:
1. 首先,你需要找到给定整数 `n` 的所有质因数。可以使用欧几里得算法(辗转相除法)来找到 `n` 和较小的素数的最大公约数(GCD),直到 `n` 变成 1。
```cpp
#include <iostream>
using namespace std;
// Function to find GCD of two numbers
int gcd(int a, int b) {
if (b == 0)
return a;
return gcd(b, a % b);
}
// Function to get prime factors
void primeFactors(int n) {
for (int i = 2; i <= sqrt(n); i++) {
while (n % i == 0) {
cout << i << " ";
n /= i; // Divide n by i until it's no longer divisible
}
}
if (n > 1) // If n is still greater than 1 after division, it's a prime factor itself
cout << n << endl;
}
```
2. 然后,对于每个质因数 `p`,计算 `log_p(n)` 来查看 `n` 是否可以表示为 `p` 的整数次幂。如果存在正整数 `k` 使得 `n = p^k`,那么 `log_p(n)` 应该是一个整数。你可以使用 C++ 标准库中的 `std::log` 函数,并结合 `std::ldexp` 函数来进行对数运算。
```cpp
#include <cmath>
// Function to check if n is a power of p (excluding p)
bool isPowerOfPrime(int n, int p) {
double log_n = log(n) / log(p);
return floor(log_n) == log_n && log_n != std::numeric_limits<double>::infinity();
}
int main() {
int n = 81; // The number you want to test
primeFactors(n);
for (int factor : factors) {
if (isPowerOfPrime(n, factor)) {
cout << n << " is a power of " << factor << endl;
}
}
return 0;
}
```
在这个例子中,`factors` 存储了 `primeFactors()` 函数返回的所有质因数。然后遍历这些质因数,对每个质因数调用 `isPowerOfPrime()` 函数来检查。
阅读全文
相关推荐
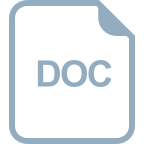
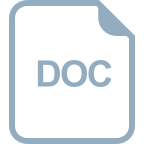















