osg如何修改模型的透明度,写一个例子出来
时间: 2024-02-27 10:54:44 浏览: 235
好的,以下是一个osg修改模型透明度的示例代码,您可以参考一下:
```cpp
#include <osg/Geode>
#include <osg/Geometry>
#include <osg/StateSet>
#include <osg/BlendFunc>
osg::ref_ptr<osg::Node> createModel()
{
// 创建一个立方体模型
osg::ref_ptr<osg::Box> box = new osg::Box(osg::Vec3(0, 0, 0), 2.0f);
osg::ref_ptr<osg::ShapeDrawable> drawable = new osg::ShapeDrawable(box);
osg::ref_ptr<osg::Geode> geode = new osg::Geode();
geode->addDrawable(drawable.get());
return geode.get();
}
int main(int argc, char** argv)
{
// 创建场景根节点
osg::ref_ptr<osg::Group> root = new osg::Group();
// 创建模型节点
osg::ref_ptr<osg::Node> model = createModel();
// 将模型节点添加到根节点中
root->addChild(model.get());
// 获取模型的状态集
osg::StateSet* stateSet = model->getOrCreateStateSet();
// 创建混合函数
osg::ref_ptr<osg::BlendFunc> blendFunc = new osg::BlendFunc();
blendFunc->setFunction(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA);
// 将混合函数添加到状态集中
stateSet->setAttributeAndModes(blendFunc.get());
// 设置透明度为半透明
float alpha = 0.5f;
osg::ref_ptr<osg::Material> material = new osg::Material();
material->setAlpha(osg::Material::FRONT, alpha);
stateSet->setAttributeAndModes(material.get());
// 创建渲染窗口
osg::ref_ptr<osgViewer::Viewer> viewer = new osgViewer::Viewer();
viewer->setUpViewInWindow(50, 50, 800, 600);
viewer->setSceneData(root.get());
// 运行主循环
return viewer->run();
}
```
该示例中,首先创建了一个立方体模型,并将其添加到场景根节点中。然后获取了模型的状态集,创建了一个混合函数,并将混合函数添加到状态集中。接着创建了一个材质对象,并设置透明度为半透明,并将其添加到状态集中。最后创建了一个渲染窗口,并运行主循环。
需要注意的是,在实际的场景中,可能还需要考虑纹理贴图、光照等因素对透明度的影响,具体实现方式还需要根据场景需求进行调整。
阅读全文
相关推荐
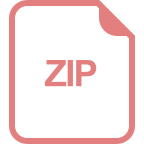
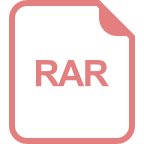
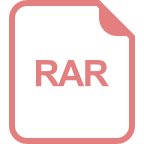
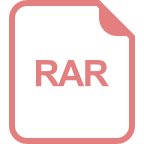
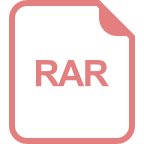
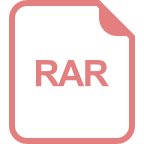
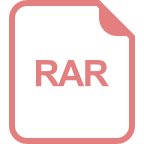









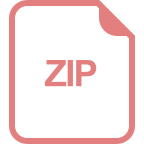