用C语言实现栈实现后缀表达式
时间: 2024-05-04 14:17:32 浏览: 15
好的,我来解答你的问题。
以下是使用C语言实现栈实现后缀表达式的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
#define MAX_STACK_SIZE 100
typedef struct {
int top;
int stack[MAX_STACK_SIZE];
} Stack;
void push(Stack *stack, int value) {
if (stack->top == MAX_STACK_SIZE) {
printf("Error: Stack is full\n");
return;
}
stack->stack[++stack->top] = value;
}
int pop(Stack *stack) {
if (stack->top == -1) {
printf("Error: Stack is empty\n");
return -1;
}
return stack->stack[stack->top--];
}
int evaluate(char *expression) {
Stack stack;
stack.top = -1;
char *token = strtok(expression, " ");
while (token != NULL) {
if (isdigit(token[0])) {
push(&stack, atoi(token));
} else {
int operand2 = pop(&stack);
int operand1 = pop(&stack);
switch (token[0]) {
case '+':
push(&stack, operand1 + operand2);
break;
case '-':
push(&stack, operand1 - operand2);
break;
case '*':
push(&stack, operand1 * operand2);
break;
case '/':
push(&stack, operand1 / operand2);
break;
default:
printf("Error: Invalid operator %c\n", token[0]);
return -1;
}
}
token = strtok(NULL, " ");
}
if (stack.top != 0) {
printf("Error: Invalid expression\n");
return -1;
}
return pop(&stack);
}
int main() {
char expression[] = "6 3 / 2 4 * +";
int result = evaluate(expression);
printf("%d\n", result);
return 0;
}
```
在上面的代码中,我们定义了一个`Stack`结构体,其中包含一个整型数组`stack`和一个整型变量`top`,用来表示栈顶元素的位置。`push`函数用来将元素压入栈中,`pop`函数用来弹出栈顶元素。
在`evaluate`函数中,我们使用`strtok`函数将后缀表达式按照空格分割成多个字符串,然后依次处理每个字符串。如果字符串是数字,就将其转换成整数并压入栈中;如果字符串是运算符,就从栈中弹出两个操作数,进行计算,并将结果压入栈中。最终,栈中只剩下一个元素,就是计算结果。
在`main`函数中,我们定义了一个后缀表达式`6 3 / 2 4 * +`,并调用`evaluate`函数计算其结果。
希望这个答案能够对你有所帮助!
相关推荐
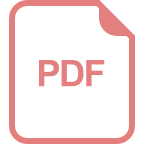














