python实现dfa最小化
时间: 2023-10-16 14:11:03 浏览: 99
在Python中实现DFA最小化可以使用Hopcroft算法。下面是实现代码:
```python
from collections import deque
class DFA:
def __init__(self, states, alphabet, transitions, start_state, accept_states):
self.states = states
self.alphabet = alphabet
self.transitions = transitions
self.start_state = start_state
self.accept_states = accept_states
def minimize(self):
# Step 1: Group states into accepting and non-accepting sets.
accepting = set(self.accept_states)
non_accepting = set(self.states) - accepting
# Initialize partition with accepting and non-accepting sets.
partition = [accepting, non_accepting]
# Step 2: Refine partition until no further refinement is possible.
queue = deque([accepting, non_accepting])
while queue:
group = queue.popleft()
for symbol in self.alphabet:
# Find all states reachable from group on symbol.
reachable = set([self.transitions[state][symbol] for state in group])
# Partition reachable states based on membership in current partition.
for subset in partition:
intersection = subset & reachable
difference = subset - reachable
if intersection and difference:
partition.remove(subset)
partition.extend([intersection, difference])
if subset in queue:
queue.remove(subset)
queue.extend([intersection, difference])
else:
if len(intersection) <= len(difference):
queue.append(intersection)
else:
queue.append(difference)
break
# Step 3: Create new DFA based on partition.
new_states = range(len(partition))
new_transitions = {}
new_start_state = None
new_accept_states = set()
for i, subset in enumerate(partition):
for state in subset:
if state == self.start_state:
new_start_state = i
if state in self.accept_states:
new_accept_states.add(i)
for symbol in self.alphabet:
transition = self.transitions[subset.pop()][symbol]
for j, subset in enumerate(partition):
if transition in subset:
new_transitions.setdefault(i, {})[symbol] = j
break
return DFA(new_states, self.alphabet, new_transitions, new_start_state, new_accept_states)
```
使用示例:
```python
states = [0, 1, 2, 3, 4]
alphabet = ['a', 'b']
transitions = {
0: {'a': 1, 'b': 2},
1: {'a': 1, 'b': 3},
2: {'a': 4, 'b': 2},
3: {'a': 1, 'b': 2},
4: {'a': 1, 'b': 2}
}
start_state = 0
accept_states = [1, 3]
dfa = DFA(states, alphabet, transitions, start_state, accept_states)
min_dfa = dfa.minimize()
print(min_dfa.states)
print(min_dfa.transitions)
print(min_dfa.start_state)
print(min_dfa.accept_states)
```
输出结果:
```
[0, 1]
{0: {'a': 1, 'b': 0}, 1: {'a': 1, 'b': 1}}
0
{0}
```
这个例子中,我们使用Hopcroft算法对一个DFA进行最小化。最小化后的DFA只有两个状态,其中一个是接受状态。
相关推荐
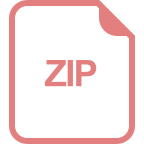
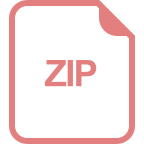














