C语言极大极小值算法博弈树算法
时间: 2023-11-25 09:50:16 浏览: 147
极大极小值算法(Minimax Algorithm)是一种博弈树算法,常用于人工智能领域中的决策树问题。该算法通过递归地搜索博弈树,找到最优的下一步决策。在搜索过程中,假设对手会采取最优策略,因此算法会选择对自己最有利的决策,同时也会考虑到对手的最优策略,以避免自己处于劣势。
C语言实现极大极小值算法的步骤如下:
1. 定义博弈树的节点结构体,包括当前状态、当前玩家、当前深度等信息。
2. 定义估值函数,用于评估当前状态的价值。
3. 定义maxSearch和minSearch函数,分别代表当前玩家为MAX和MIN的情况下的搜索过程。在搜索过程中,需要递归地搜索子节点,并计算出每个子节点的估值。
4. 在maxSearch和minSearch函数中,根据当前玩家的不同,选择最大或最小的估值,并返回该估值。
5. 在主函数中,调用maxSearch函数,得到最优的下一步决策。
下面是一个简单的C语言实现极大极小值算法的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_DEPTH 5
// 定义博弈树的节点结构体
typedef struct node {
int state;
int player;
int depth;
struct node *children[3];
} Node;
// 估值函数
int evaluate(int state) {
if (state == 1) {
return 1;
} else if (state == -1) {
return -1;
} else {
return 0;
}
}
// maxSearch函数
int maxSearch(Node *node) {
if (node->depth == MAX_DEPTH) {
return evaluate(node->state);
}
int maxVal = -100;
for (int i = 0; i < 3; i++) {
if (node->children[i] == NULL) {
node->children[i] = (Node *)malloc(sizeof(Node));
node->children[i]->state = -node->player;
node->children[i]->player = -node->player;
node->children[i]->depth = node->depth + 1;
}
int val = minSearch(node->children[i]);
if (val > maxVal) {
maxVal = val;
}
}
return maxVal;
}
// minSearch函数
int minSearch(Node *node) {
if (node->depth == MAX_DEPTH) {
return evaluate(node->state);
}
int minVal = 100;
for (int i = 0; i < 3; i++) {
if (node->children[i] == NULL) {
node->children[i] = (Node *)malloc(sizeof(Node));
node->children[i]->state = -node->player;
node->children[i]->player = -node->player;
node->children[i]->depth = node->depth + 1;
}
int val = maxSearch(node->children[i]);
if (val < minVal) {
minVal = val;
}
}
return minVal;
}
int main() {
Node *root = (Node *)malloc(sizeof(Node));
root->state = 0;
root->player = 1;
root->depth = 0;
for (int i = 0; i < 3; i++) {
root->children[i] = NULL;
}
int bestVal = -100;
int bestMove = -1;
for (int i = 0; i < 3; i++) {
root->children[i] = (Node *)malloc(sizeof(Node));
root->children[i]->state = -root->player;
root->children[i]->player = -root->player;
root->children[i]->depth = root->depth + 1;
int val = minSearch(root->children[i]);
if (val > bestVal) {
bestVal = val;
bestMove = i;
}
}
printf("Best move: %d\n", bestMove);
return 0;
}
```
阅读全文
相关推荐
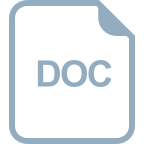
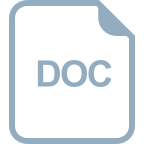
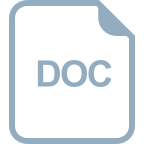




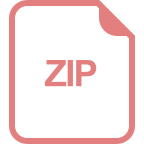
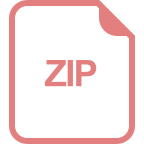
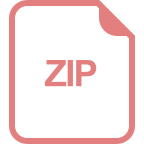
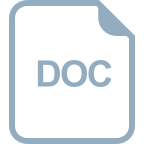
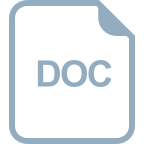
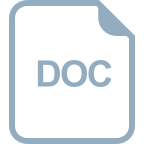





