modbus.c代码
时间: 2024-04-30 09:16:45 浏览: 102
modbus.c是一个用于实现Modbus通信协议的C语言代码文件。Modbus是一种常用的串行通信协议,用于在工业自动化领域中实现设备之间的通信。
在modbus.c代码中,通常包含了以下功能:
1. 初始化Modbus通信:包括设置串口参数、建立连接等。
2. 发送Modbus请求:根据Modbus协议格式,构建并发送请求帧给目标设备。
3. 接收Modbus响应:监听串口,接收目标设备返回的响应帧。
4. 解析Modbus响应:根据Modbus协议格式,解析响应帧中的数据。
5. 处理Modbus异常:处理目标设备返回的异常响应。
具体的modbus.c代码实现可能会根据不同的应用场景和设备要求有所不同。你可以提供更具体的问题或者需求,我可以给出更详细的回答。
相关问题
stm32 modbus.c
STM32 Modbus.c是一种用于STM32微控制器的Modbus通信协议实现源码。Modbus是一种常用的工业通信协议,用于不同设备之间的通信和数据交换。
STM32 Modbus.c提供了一个可编程的用于STM32微控制器的Modbus通信协议库。通过该库,开发人员可以在STM32平台上实现Modbus通信功能,以便与其他Modbus兼容设备进行通信。
该库中的Modbus.c文件实现了Modbus协议的相关功能,包括数据包的封装和解析、CRC校验、功能代码的支持等等。通过使用该源码,用户可以快速开发自己的Modbus通信应用。用户只需要根据自己的需求修改和扩展源码,即可实现不同功能、不同设备之间的Modbus通信。
在STM32 Modbus.c源码中,通信协议的相关细节已经被封装起来,开发人员只需要调用相应的函数,即可实现与其他Modbus设备的通信。该源码可运行在STM32微控制器上,具有良好的稳定性和高效性能。
总之,STM32 Modbus.c提供了一个方便实用的Modbus通信协议实现源码,使得开发人员可以在STM32平台上轻松实现Modbus通信功能,并与其他Modbus兼容设备进行数据交换。
modbus的C语言代码
以下是一个简单的用C语言实现Modbus RTU通信的示例代码,用于读取一个保持寄存器的值:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <termios.h>
#include <unistd.h>
#include <fcntl.h>
#include <errno.h>
#define SLAVE_ADDRESS 1
#define FUNCTION_READ_HOLDING_REGISTERS 0x03
#define REGISTER_ADDRESS 0x0000
#define DATA_LENGTH 0x0001
unsigned short crc16(unsigned char *buf, int len) {
unsigned short crc = 0xFFFF;
int i, j;
for (i = 0; i < len; i++) {
crc ^= buf[i];
for (j = 0; j < 8; j++) {
if (crc & 0x0001) {
crc >>= 1;
crc ^= 0xA001;
} else {
crc >>= 1;
}
}
}
return crc;
}
int main(int argc, char *argv[]) {
int fd;
struct termios options;
if (argc < 2) {
printf("Usage: %s /dev/ttyUSB0\n", argv[0]);
return 1;
}
fd = open(argv[1], O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1) {
perror("open");
return 1;
}
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag &= ~CRTSCTS;
options.c_iflag &= ~(IXON | IXOFF | IXANY);
options.c_iflag &= ~(ICANON | ECHO | ECHOE | ISIG);
options.c_oflag &= ~OPOST;
options.c_cc[VMIN] = 1;
options.c_cc[VTIME] = 0;
tcsetattr(fd, TCSANOW, &options);
unsigned char request[8];
request[0] = SLAVE_ADDRESS;
request[1] = FUNCTION_READ_HOLDING_REGISTERS;
request[2] = REGISTER_ADDRESS >> 8;
request[3] = REGISTER_ADDRESS & 0xFF;
request[4] = DATA_LENGTH >> 8;
request[5] = DATA_LENGTH & 0xFF;
unsigned short crc = crc16(request, 6);
request[6] = crc & 0xFF;
request[7] = crc >> 8;
write(fd, request, 8);
unsigned char response[5 + 2 * DATA_LENGTH];
int n = read(fd, response, 5 + 2 * DATA_LENGTH);
if (n < 5 + 2 * DATA_LENGTH) {
printf("Error: Modbus response truncated\n");
return 1;
}
crc = crc16(response, 3 + 2 * DATA_LENGTH);
if (crc != (response[3 + 2 * DATA_LENGTH] + (response[4 + 2 * DATA_LENGTH] << 8))) {
printf("Error: Modbus response CRC error\n");
return 1;
}
if (response[1] & 0x80) {
printf("Error: Modbus response exception code %02X\n", response[2]);
return 1;
}
unsigned short result = (response[3] << 8) + response[4];
printf("Result: %d\n", result);
close(fd);
return 0;
}
```
这个代码使用Linux系统提供的串口接口进行通信,需要按照实际情况修改串口设备文件名和波特率。在程序中,首先设置串口参数,然后构造一个读取保持寄存器的请求,发送到Modbus从机上,并等待响应。最后,检查响应是否有效,如果没有错误,则将结果打印出来。
阅读全文
相关推荐
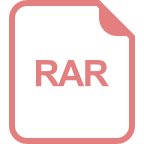
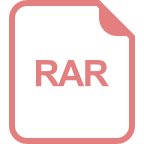
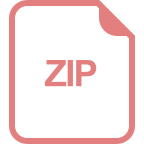
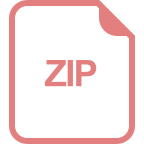
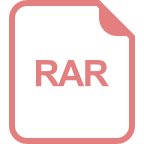
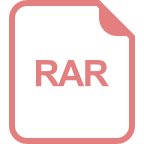
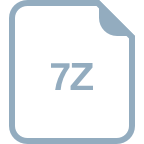
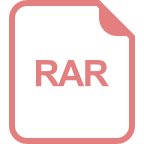
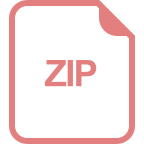
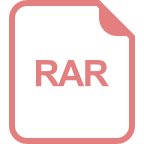
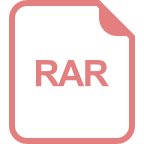
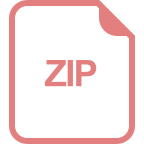
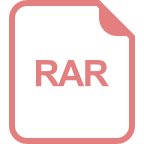