C# debug.log
时间: 2024-09-28 08:11:58 浏览: 46
在C#中,并没有直接称为"debug.log"的概念,它不是C#语言内置的日志文件功能。通常开发者会使用.NET Framework提供的System.Diagnostics命名空间下的日志类如`System.Diagnostics.Trace`、`Console.WriteLine`、`File.WriteAllText`等来进行程序运行时的调试信息记录。
例如,你可以创建一个名为`log.txt`的文件,在需要记录日志的地方使用以下代码:
```csharp
using System.IO;
public static void LogInfo(string message)
{
string logFilePath = "log.txt";
File.AppendAllText(logFilePath, $"{DateTime.Now}: {message}\n");
}
```
然后在你的代码中通过`LogInfo("This is a debug message.");`来添加一条记录。如果你想要更详细的控制,还可以使用`Trace.WriteLine`配合`Trace.Listeners`来配置自定义的监听器,比如文件监听器。
相关问题
举个C#debug.log的例子
在C#语言中没有内置的 `debug.log()` 函数,但可以使用 `System.Diagnostics.Debug` 类中的方法来实现。下面是一个简单的例子:
```
using System.Diagnostics;
class Program
{
static void Main(string[] args)
{
int x = 10;
// 输出调试信息
Debug.WriteLine("x的值为:" + x);
// 等价于
// Debug.WriteLine($"x的值为:{x}");
}
}
```
在上述代码中,我们首先使用 `using System.Diagnostics;` 导入了 `System.Diagnostics` 命名空间。然后定义了一个变量 `x`,并使用 `Debug.WriteLine()` 方法输出了它的值。当我们运行这段代码时,如果设置了调试输出,就可以在输出窗口上看到类似下面的输出:
```
x的值为:10
```
这样,我们就可以方便地输出程序中关键变量的值,从而更好地理解程序的执行过程和调试错误。需要注意的是,这种方式输出的调试信息只在调试模式下才会显示,发布版本不会输出。
using System.Collections; using System.Collections.Generic; using UnityEngine; public class MatSwap : MonoBehaviour { public Material originalMaterial; public GameObject obj; void Start() { Material clonedMaterial = new Material (originalMaterial); Renderer renderer = obj.GetComponent<Renderer>(); renderer.material = clonedMaterial; foreach (Material material in obj) { Debug.Log(material.name); Debug.Log(material.color); } } }
这段代码定义了一个名为MatSwap的C#脚本,它包含了一个公共的材质originalMaterial和一个公共的游戏对象obj。在Start函数中,它创建了一个名为clonedMaterial的材质副本,并将其赋值给游戏对象的渲染器组件的材质属性。最后,它使用foreach循环遍历游戏对象的所有材质,并输出每个材质的名称和颜色到控制台。这段代码的作用是在运行时将游戏对象的材质替换为原始材质的副本,并打印所有材质的名称和颜色。
阅读全文
相关推荐
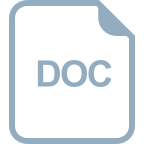
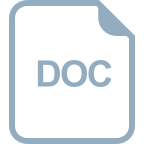
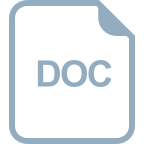


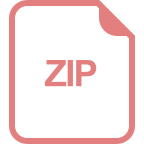
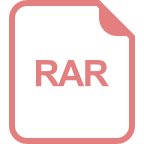







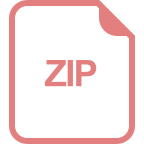
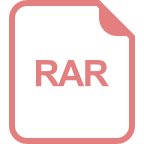
