使用pytorch进行图像分割的代码
时间: 2023-05-22 11:04:47 浏览: 251
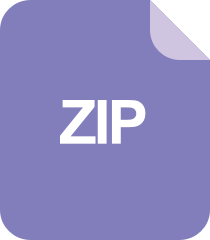
基于pytorch实现segnet的图像分割任务python源码.zip
可以在PyTorch官方文档和GitHub仓库中找到许多使用PyTorch进行图像分割的代码。以下是一个例子,使用的是PyTorch中的FCN模型,以VOC2012的分割任务为例:
```python
import torch
import torch.nn as nn
import torchvision.models as models
class FCN8s(nn.Module):
def __init__(self, num_classes):
super(FCN8s, self).__init__()
# Load a pre-trained VGG16 model from torchvision.models
vgg16 = models.vgg16(pretrained=True)
# Only keep the first 10 convolutional layers, freeze them and replace the 7th to
# 10th convolutional layers with corresponding upsampling layers
features, classifier = list(vgg16.features.children()), list(vgg16.classifier.children())
features = nn.Sequential(*features)[:10]
for layer in features:
for parameter in layer.parameters():
parameter.requires_grad_(False)
classifier = nn.Sequential(
nn.Conv2d(512, 4096, kernel_size=7),
nn.ReLU(inplace=True),
nn.Dropout(),
nn.Conv2d(4096, 4096, kernel_size=1),
nn.ReLU(inplace=True),
nn.Dropout(),
nn.Conv2d(4096, num_classes, kernel_size=1),
)
# Set the output stride of the model to be 8 by inserting an upsampling layer after
# the 5th max pooling layer
features[4] = nn.Conv2d(512, 512, kernel_size=3, padding=1)
classifier = nn.Sequential(
*classifier,
nn.ConvTranspose2d(num_classes, num_classes, kernel_size=4, stride=2, bias=False),
nn.ReLU(inplace=True),
nn.ConvTranspose2d(num_classes, num_classes, kernel_size=4, stride=2, bias=False),
nn.ReLU(inplace=True),
nn.ConvTranspose2d(num_classes, num_classes, kernel_size=16, stride=8, bias=False),
)
# Define the final FCN model by combining the modified VGG16 model with the classifier
self.features = features
self.classifier = classifier
def forward(self, x):
x = self.features(x)
x = self.classifier(x)
return x
# Example usage
fcn = FCN8s(num_classes=21)
input_tensor = torch.randn(1, 3, 224, 224)
output_tensor = fcn(input_tensor)
print(output_tensor.shape)
```
以上代码定义了一个FCN8s模型,使用了预训练的VGG16作为特征提取器,用于将输入图像的像素特征映射到一个低维的语义特征空间。在特征提取器的基础上,添加一个卷积分类器用于将语义特征映射到实际的分割结果,最后通过叠加三个反卷积层进行上采样,得到分割结果。输入图像的尺寸为`(batch_size, 3, height, width)`,输出分割结果的尺寸为`(batch_size, num_classes, out_height, out_width)`。
注:这里只是举一个例子说明如何使用PyTorch进行图像分割,具体实现还需要根据不同的数据集和任务进行调整和修改。
阅读全文
相关推荐
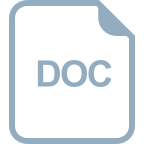
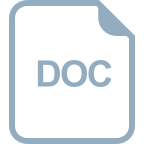
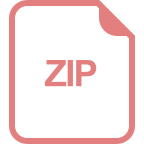
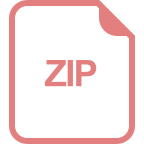
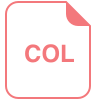



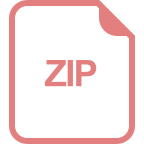
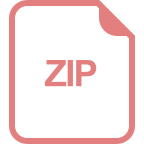
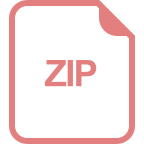
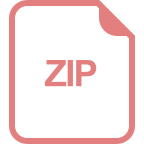
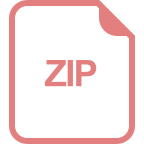
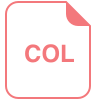
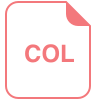


