用c++类与对象写一个贪吃蛇游戏
时间: 2023-08-04 09:28:58 浏览: 77
好的,让我们来写一个简单的贪吃蛇游戏。
首先,我们需要定义一个蛇类(Snake),该类应该包含以下属性和方法:
属性:
- vector<pair<int, int>> body:蛇身体的坐标,由许多二元组构成
方法:
- void move(int direction):根据方向移动蛇
- bool eat(pair<int, int> food):判断蛇是否吃到了食物,如果吃到了,返回 true,否则返回 false
- bool isDead():判断蛇是否死亡,如果死亡,返回 true,否则返回 false
接下来,我们需要定义一个游戏类(Game),该类应该包含以下属性和方法:
属性:
- int width:游戏区域的宽度
- int height:游戏区域的高度
- pair<int, int> food:食物的坐标
- Snake snake:蛇的实例
方法:
- void init():初始化游戏,包括创建蛇和放置食物
- void run():运行游戏,处理用户输入和每一帧的逻辑
- void draw():绘制游戏界面
下面是完整的代码实现:
```cpp
#include <iostream>
#include <vector>
#include <stdlib.h>
#include <conio.h>
#include <Windows.h>
using namespace std;
class Snake {
public:
vector<pair<int, int>> body;
Snake(int x, int y) {
body.push_back(make_pair(x, y));
}
void move(int direction) {
int dx, dy;
switch (direction) {
case 0: // up
dx = -1; dy = 0;
break;
case 1: // right
dx = 0; dy = 1;
break;
case 2: // down
dx = 1; dy = 0;
break;
case 3: // left
dx = 0; dy = -1;
break;
}
for (int i = body.size() - 1; i > 0; i--) {
body[i].first = body[i - 1].first;
body[i].second = body[i - 1].second;
}
body[0].first += dx;
body[0].second += dy;
}
bool eat(pair<int, int> food) {
if (body[0].first == food.first && body[0].second == food.second) {
body.push_back(food);
return true;
}
return false;
}
bool isDead(int width, int height) {
if (body[0].first < 0 || body[0].first >= height || body[0].second < 0 || body[0].second >= width) {
return true;
}
for (int i = 1; i < body.size(); i++) {
if (body[i].first == body[0].first && body[i].second == body[0].second) {
return true;
}
}
return false;
}
};
class Game {
public:
int width, height;
pair<int, int> food;
Snake snake;
Game(int w, int h) : width(w), height(h), snake(w / 2, h / 2) {
init();
}
void init() {
srand((unsigned)time(NULL));
food.first = rand() % height;
food.second = rand() % width;
}
void run() {
int direction = 1;
while (true) {
if (_kbhit()) {
int key = _getch();
if (key == 224) {
key = _getch();
switch (key) {
case 72: // up
direction = 0;
break;
case 77: // right
direction = 1;
break;
case 80: // down
direction = 2;
break;
case 75: // left
direction = 3;
break;
}
}
}
snake.move(direction);
if (snake.eat(food)) {
food.first = rand() % height;
food.second = rand() % width;
}
if (snake.isDead(width, height)) {
break;
}
draw();
Sleep(100);
}
}
void draw() {
system("cls");
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
if (i == food.first && j == food.second) {
cout << "@";
}
else {
bool flag = false;
for (int k = 0; k < snake.body.size(); k++) {
if (snake.body[k].first == i && snake.body[k].second == j) {
cout << "O";
flag = true;
break;
}
}
if (!flag) {
cout << " ";
}
}
}
cout << endl;
}
}
};
int main() {
Game game(20, 20);
game.run();
return 0;
}
```
这个代码可能有点长,但是它很好理解。我们在游戏类中使用了 Snake 类,通过绘制和处理用户输入来控制蛇的移动和生死。你可以尝试运行这个代码,并根据自己的需要进行修改和完善。
阅读全文
相关推荐
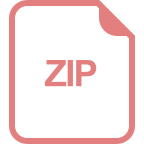
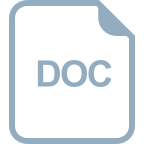
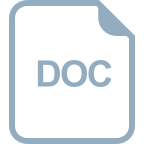
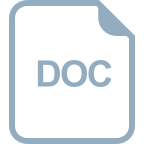
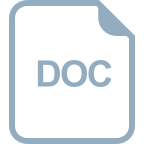







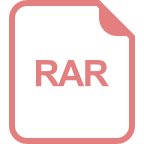
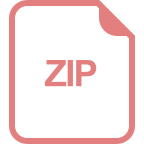
