无迹卡尔曼滤波 python代码
时间: 2024-04-17 15:22:29 浏览: 27
无迹卡尔曼滤波(Unscented Kalman Filter,UKF)是一种非线性滤波算法,用于估计系统状态。下面是一个简单的无迹卡尔曼滤波的Python代码示例:
```python
import numpy as np
def unscented_kalman_filter(x, P, z, Q, R, f, h):
n = len(x)
m = len(z)
alpha = 0.001
kappa = 0
beta = 2
lambda_ = alpha**2 * (n + kappa) - n
c = n + lambda_
# Generate sigma points
sigma_points = np.zeros((n, 2*n+1))
sigma_points[:, 0] = x
sqrt_P = np.linalg.cholesky(c*P)
for i in range(n):
sigma_points[:, i+1] = x + sqrt_P[:, i]
sigma_points[:, n+i+1] = x - sqrt_P[:, i]
# Propagate sigma points through the nonlinear process model
predicted_sigma_points = np.zeros((n, 2*n+1))
for i in range(2*n+1):
predicted_sigma_points[:, i] = f(sigma_points[:, i])
# Calculate predicted state and covariance
x_pred = np.sum(predicted_sigma_points, axis=1) / c
P_pred = np.zeros((n, n))
for i in range(2*n+1):
diff = predicted_sigma_points[:, i] - x_pred
P_pred += (diff.reshape(-1, 1) @ diff.reshape(1, -1)) / c
P_pred += Q
# Calculate predicted measurement and covariance
predicted_measurements = np.zeros((m, 2*n+1))
for i in range(2*n+1):
predicted_measurements[:, i] = h(predicted_sigma_points[:, i])
z_pred = np.sum(predicted_measurements, axis=1) / c
Pz_pred = np.zeros((m, m))
for i in range(2*n+1):
diff = predicted_measurements[:, i] - z_pred
Pz_pred += (diff.reshape(-1, 1) @ diff.reshape(1, -1)) / c
Pz_pred += R
# Calculate cross-covariance matrix
Pxz = np.zeros((n, m))
for i in range(2*n+1):
x_diff = predicted_sigma_points[:, i] - x_pred
z_diff = predicted_measurements[:, i] - z_pred
Pxz += (x_diff.reshape(-1, 1) @ z_diff.reshape(1, -1)) / c
# Calculate Kalman gain
K = Pxz @ np.linalg.inv(Pz_pred)
# Update state and covariance
x = x_pred + K @ (z - z_pred)
P = P_pred - K @ Pz_pred @ K.T
return x, P
# 定义非线性过程模型函数 f(x)
def process_model(x):
return np.array([x + x, x])
# 定义非线性测量模型函数 h(x)
def measurement_model(x):
return np.array([x])
# 初始化状态和协方差矩阵
x = np.array([0, 0])
P = np.eye(2)
# 测量噪声协方差矩阵
R = np.array([[0.1]])
# 过程噪声协方差矩阵
Q = np.array([[0.01, 0], [0, 0.01]])
# 测量值
z = np.array()
# 使用无迹卡尔曼滤波进行状态估计
x_est, P_est = unscented_kalman_filter(x, P, z, Q, R, process_model, measurement_model)
print("Estimated state:", x_est)
print("Estimated covariance:", P_est)
```
相关推荐
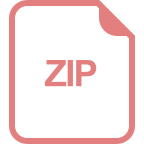
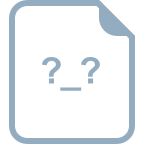













