在单链表末尾插入元素生成{1,3,5,7},给出C++代码
时间: 2024-12-08 17:22:55 浏览: 8
在单链表的末尾插入元素生成一个等差数列{1,3,5,7},我们可以先创建一个头指针作为链表的起点,然后遍历链表并在每个节点之后插入新的值。由于这是一个等差序列,我们可以直接计算新节点的位置。
以下是C++代码示例:
```cpp
#include <iostream>
// 定义链表节点结构体
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(nullptr) {}
};
// 在链表末尾插入新节点并返回链表头指针
ListNode* insertAtEnd(ListNode* head, int n, int lastNum = 0) {
if (!head) { // 如果链表为空,直接创建第一个节点
return new ListNode(n);
}
ListNode* newNode = new ListNode(n); // 新节点值
ListNode* current = head; // 当前遍历到的节点
while (current->next != nullptr && current->val + 2 <= lastNum) {
current = current->next; // 跳过已存在的节点
}
// 插入新节点
newNode->next = current->next;
current->next = newNode;
return head;
}
// 打印链表
void printList(ListNode* head) {
ListNode* temp = head;
while (temp) {
std::cout << temp->val << " ";
temp = temp->next;
}
std::cout << "\n";
}
int main() {
ListNode* head = nullptr;
for (int i = 1; i <= 4; ++i) { // 生成1, 3, 5, 7
head = insertAtEnd(head, i * 2 - 1);
}
std::cout << "Final linked list: ";
printList(head);
return 0;
}
```
在这个例子中,`insertAtEnd`函数负责在合适的位置插入新节点,然后`main`函数用于测试这个功能。运行这段代码后,将得到一个按顺序排列的链表:1, 3, 5, 7。
阅读全文
相关推荐
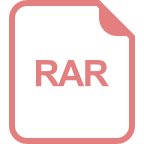
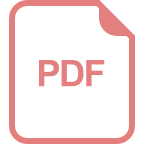
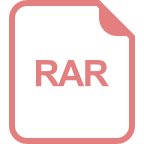















