qt 求圆与直线的交点
时间: 2024-05-18 17:08:42 浏览: 19
在Qt中,可以使用数学库来计算圆与直线的交点。首先,需要确定圆和直线的方程。假设圆的方程为(x-a)^2 + (y-b)^2 = r^2,其中(a, b)为圆心坐标,r为半径;直线的方程为y = kx + c,其中k为斜率,c为截距。
要求圆与直线的交点,可以将直线方程代入圆的方程,得到一个关于x的二次方程。解这个二次方程可以得到x的两个解,再将这两个解代入直线方程,即可得到对应的y值。
以下是一个示例代码,演示了如何在Qt中计算圆与直线的交点:
```cpp
#include <QCoreApplication>
#include <QDebug>
#include <cmath>
struct Point {
double x;
double y;
};
QVector<Point> calculateIntersection(double a, double b, double r, double k, double c) {
QVector<Point> intersections;
// 计算二次方程的系数
double A = 1 + k * k;
double B = -2 * a + 2 * k * (c - b);
double C = a * a + (c - b) * (c - b) - r * r;
// 计算判别式
double discriminant = B * B - 4 * A * C;
if (discriminant >= 0) {
// 计算交点的x坐标
double x1 = (-B + std::sqrt(discriminant)) / (2 * A);
double x2 = (-B - std::sqrt(discriminant)) / (2 * A);
// 计算交点的y坐标
double y1 = k * x1 + c;
double y2 = k * x2 + c;
intersections.append({x1, y1});
intersections.append({x2, y2});
}
return intersections;
}
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
double circleCenterX = 0;
double circleCenterY = 0;
double radius = 5;
double lineSlope = 1;
double lineIntercept = 2;
QVector<Point> intersections = calculateIntersection(circleCenterX, circleCenterY, radius, lineSlope, lineIntercept);
qDebug() << "Intersection points:";
for (const Point& point : intersections) {
qDebug() << "x:" << point.x << ", y:" << point.y;
}
return a.exec();
}
```
请注意,上述代码仅为示例,实际使用时需要根据具体情况进行适当修改。另外,需要包含Qt的头文件和使用Qt的数据结构,如QVector和QDebug。
相关推荐
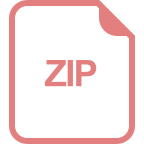
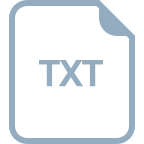
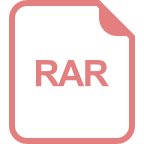














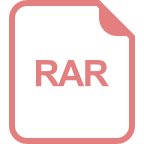