cpp代码使用Bresenham算法实现直线扫描转换,要求可以显示出来一个坐标轴显示结果的点集
时间: 2024-10-18 10:13:55 浏览: 40
在C++中,我们可以使用Bresenham算法来绘制一条直线并显示在坐标轴上。这里是一个简单的示例,假设我们有一个二维数组`grid`表示屏幕,我们将用`x`和`y`作为输入的起始和结束点:
```cpp
#include <iostream>
#include <vector>
// Bresenham's Line Algorithm
std::vector<std::pair<int, int>> bresenham(int x0, int y0, int x1, int y1) {
std::vector<std::pair<int, int>> points;
int dx = std::abs(x1 - x0);
int dy = std::abs(y1 - y0);
bool steep = dy > dx;
if (steep) {
std::swap(x0, y0);
std::swap(x1, y1);
}
int error = steep ? dx : -dy;
int y = steep ? y0 : y0;
for (int x = x0; x <= x1; x++) {
if (steep) {
points.push_back({y, x});
} else {
points.push_back({x, y});
}
error += dy;
if (error >= 0) {
y += steep ? 1 : 1;
error -= dx;
}
}
return points;
}
void print_points_on_axis(std::vector<std::pair<int, int>>& points, int width, int height) {
// Draw points on the grid (assuming a console-based output)
for (const auto& point : points) {
int row = point.first;
int col = point.second;
// Adjust indices to fit in the coordinate system (if needed)
row = height - row - 1;
col = col % width; // Wrap around when reaching edge
// Print the point as a dot or square depending on your preference
std::cout << "· ";
}
std::cout << "\n";
}
int main() {
int x0 = 0, y0 = 0, x1 = 5, y1 = 5; // Example start and end points
std::vector<std::pair<int, int>> line_points = bresenham(x0, y0, x1, y1);
int screen_width = 10; // Size of the screen (or console display)
int screen_height = screen_width;
print_points_on_axis(line_points, screen_width, screen_height);
return 0;
}
```
在这个例子中,我们首先定义了一个Bresenham算法函数,然后创建一个`print_points_on_axis`函数来打印出在给定坐标范围内的点。最后,在`main`函数里,我们调用这两个函数并输出结果。记得调整屏幕大小(`screen_width` 和 `screen_height`)以适应实际应用环境。
运行此程序,将会在控制台上显示出一条从`(0, 0)`到`(5, 5)`的直线,并显示各个点的位置。注意,这只是一个基础的实现,实际应用中可能需要更复杂的处理,比如颜色填充等。
阅读全文
相关推荐
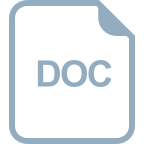
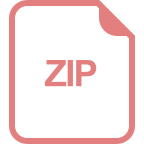
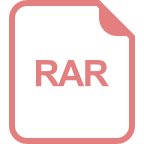

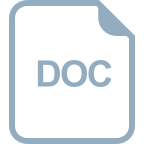




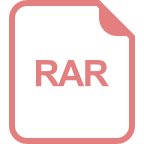
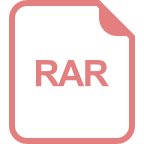
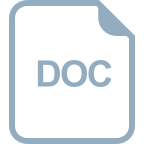
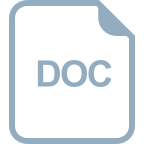
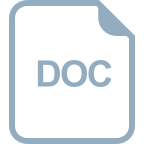
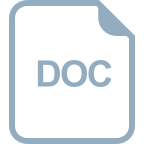



