opencv车牌识别用pytesseract
时间: 2024-12-27 17:21:55 浏览: 3
### 使用 OpenCV 和 PyTesseract 进行车牌识别
为了实现车牌识别,需要先安装必要的库。可以通过 `pip` 安装所需的软件包:
```bash
pip install opencv-python-headless pytesseract
```
#### 导入所需库
在代码中引入所有必需的包,特别是用于计算机视觉操作的 OpenCV 和作为 Tesseract OCR 引擎 Python 包装器的 PyTesseract[^3]。
```python
import cv2
import pytesseract
from PIL import Image
```
#### 图像预处理
图像预处理对于提高OCR准确性至关重要。这里展示了一个基本流程,包括灰度转换、噪声去除以及二值化处理等步骤[^1]。
```python
def preprocess_image(image_path):
img = cv2.imread(image_path, cv2.IMREAD_COLOR)
# 将图片转为灰色图
gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 应用高斯模糊减少噪音并使边缘更平滑
blurred_img = cv2.GaussianBlur(gray_img, (5, 5), 0)
# 自适应阈值处理得到二值图像
binary_img = cv2.adaptiveThreshold(blurred_img, 255, cv2.ADAPTIVE_THRESH_GAUSSIAN_C,
cv2.THRESH_BINARY_INV, 11, 2)
return binary_img
```
#### 车牌定位与提取
通过形态学变换找到可能包含车牌区域的最大矩形轮廓,并将其裁剪出来以便后续处理[^4]。
```python
def find_plate_contour(binary_img):
contours, _ = cv2.findContours(binary_img.copy(), cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
contour_areas = [(cv2.contourArea(c), c) for c in contours]
if not contour_areas:
raise ValueError('No plate found')
largest_contour = max(contour_areas, key=lambda x: x[0])[1]
rect = cv2.minAreaRect(largest_contour)
box = cv2.boxPoints(rect)
box = np.intp(box)
cropped_plate = four_point_transform(binary_img, box.reshape(4, 2))
return cropped_plate
def order_points(pts):
"""Sort the points based on their position."""
pts = np.array(pts).reshape((4, 2))
s = pts.sum(axis=1)
diff = np.diff(pts, axis=1)
ordered_pts = [
pts[np.argmin(s)], # Top-left point has smallest sum of coordinates.
pts[np.argmax(diff)], # Bottom-right point has greatest difference between its X and Y coordinate values.
pts[np.argmin(diff)], # Top-right point has least difference between its X and Y coordinate values.
pts[np.argmax(s)] # Bottom-left point has highest sum of coordinates.
]
return np.float32(ordered_pts)
def four_point_transform(image, pts):
"""Apply a perspective transform to get top-down view of an image given four points defining rectangle corners"""
rect = order_points(pts)
(tl, tr, br, bl) = rect
widthA = np.sqrt(((br[0] - bl[0]) ** 2) + ((br[1] - bl[1]) ** 2))
widthB = np.sqrt(((tr[0] - tl[0]) ** 2) + ((tr[1] - tl[1]) ** 2))
maxWidth = max(int(widthA), int(widthB))
heightA = np.sqrt(((tr[0] - br[0]) ** 2) + ((tr[1] - br[1]) ** 2))
heightB = np.sqrt(((tl[0] - bl[0]) ** 2) + ((tl[1] - bl[1]) ** 2))
maxHeight = max(int(heightA), int(heightB))
dst = np.array([
[0, 0],
[maxWidth - 1, 0],
[maxWidth - 1, maxHeight - 1],
[0, maxHeight - 1]], dtype="float32")
M = cv2.getPerspectiveTransform(rect, dst)
warped = cv2.warpPerspective(image, M, (maxWidth, maxHeight))
return warped
```
#### 字符识别
利用 PyTesseract 对经过上述处理后的图像执行光学字符识别(OCR),从而获取车牌上的文字信息[^2]。
```python
pytesseract.pytesseract.tesseract_cmd = r'path_to_tesseract_executable'
def recognize_text_from_image(processed_image):
text = pytesseract.image_to_string(Image.fromarray(processed_image), lang='eng')
cleaned_text = ''.join(e for e in text if e.isalnum())
return cleaned_text.upper()
```
#### 主函数调用
最后,在主程序里依次调用以上定义好的各个子过程完成整个车牌识别的任务逻辑。
```python
if __name__ == "__main__":
input_image_path = "sample_license_plate.jpg"
processed_image = preprocess_image(input_image_path)
try:
license_plate_region = find_plate_contour(processed_image)
recognized_text = recognize_text_from_image(license_plate_region)
print(f"The detected license plate number is {recognized_text}.")
except Exception as ex:
print(ex)
```
阅读全文
相关推荐
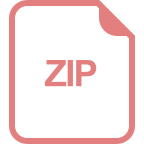
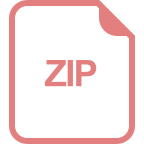
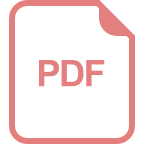
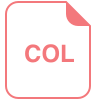
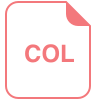
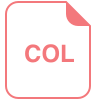












