opencv车牌识别完整代码
时间: 2023-07-31 17:12:04 浏览: 48
这是一个基于OpenCV的车牌识别完整代码,包括车牌定位、车牌字符分割和字符识别等步骤,你可以根据自己的需求进行修改和优化。
```
import cv2
import numpy as np
import pytesseract
# 车牌定位
def locate_license_plate(img):
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 高斯滤波
blur = cv2.GaussianBlur(gray, (5, 5), 0)
# Canny边缘检测
canny = cv2.Canny(blur, 50, 150)
# 轮廓检测
contours, hierarchy = cv2.findContours(canny, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# 遍历所有轮廓
for contour in contours:
# 计算轮廓面积
area = cv2.contourArea(contour)
if area < 1000: # 过滤面积较小的轮廓
continue
# 计算轮廓周长
perimeter = cv2.arcLength(contour, True)
# 多边形拟合
approx = cv2.approxPolyDP(contour, 0.02 * perimeter, True)
if len(approx) == 4: # 选取4个顶点的轮廓
x, y, w, h = cv2.boundingRect(contour)
# 过滤不符合长宽比的轮廓
if w / h > 2.5 or h / w > 2.5:
continue
# 裁剪出车牌区域
license_plate = img[y:y + h, x:x + w]
return license_plate
# 车牌字符分割
def segment_license_plate(license_plate):
gray = cv2.cvtColor(license_plate, cv2.COLOR_BGR2GRAY)
# 二值化
_, binary = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)
# 腐蚀
kernel = np.ones((3, 3), np.uint8)
erosion = cv2.erode(binary, kernel, iterations=1)
# 轮廓检测
contours, hierarchy = cv2.findContours(erosion, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
char_imgs = []
# 遍历所有轮廓
for contour in contours:
# 计算轮廓面积
area = cv2.contourArea(contour)
if area < 50: # 过滤面积较小的轮廓
continue
# 计算轮廓周长
perimeter = cv2.arcLength(contour, True)
# 多边形拟合
approx = cv2.approxPolyDP(contour, 0.02 * perimeter, True)
x, y, w, h = cv2.boundingRect(contour)
# 过滤不符合长宽比的轮廓
if w / h > 3 or h / w > 3:
continue
# 裁剪出字符图片
char_img = license_plate[y:y + h, x:x + w]
char_imgs.append(char_img)
return char_imgs
# 字符识别
def recognize_chars(char_imgs):
chars = []
# 遍历所有字符图片
for char_img in char_imgs:
# 二值化
gray = cv2.cvtColor(char_img, cv2.COLOR_BGR2GRAY)
_, binary = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)
# 调整大小
resized = cv2.resize(binary, (20, 30), interpolation=cv2.INTER_AREA)
# 转换为字符串
char = pytesseract.image_to_string(resized, config="--psm 10")
chars.append(char)
return chars
# 加载图片
img = cv2.imread("car.jpg")
# 车牌定位
license_plate = locate_license_plate(img)
# 车牌字符分割
char_imgs = segment_license_plate(license_plate)
# 字符识别
chars = recognize_chars(char_imgs)
# 显示结果
cv2.imshow("License Plate", license_plate)
for i, char in enumerate(chars):
cv2.imshow("Char %d" % i, char)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这段代码首先使用 `locate_license_plate` 函数对输入的图片进行车牌定位,然后使用 `segment_license_plate` 函数对车牌进行字符分割,最后使用 `recognize_chars` 函数对每个字符进行识别。最终会显示车牌和每个字符的识别结果。
相关推荐
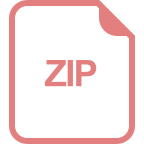
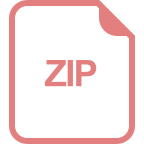













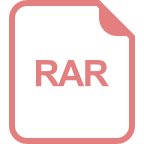