用c++编码判断两棵二叉树是否同构 要求:假设二叉树采用二叉链存储结构存放,设计一个递归算法判断两棵二叉树btl和bt2是否同构。编写完整的实验程序,并采用相应数据进行测试。
时间: 2024-10-16 21:05:28 浏览: 30
在 C++ 中,我们可以使用递归的方式来比较两棵二叉树是否同构。首先,我们需要定义两个节点结构体,分别表示两棵树的节点。然后,我们定义一个函数 `isIsomorphic` 来执行比较,这个函数会检查当前节点、其左子节点以及右子节点是否对应同构。
```cpp
#include <iostream>
using namespace std;
// 二叉树节点定义
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x) : val(x), left(NULL), right(NULL) {}
};
// 判断两个节点是否同构
bool isIsomorphic(TreeNode* node1, TreeNode* node2) {
if (node1 == nullptr && node2 == nullptr)
return true;
else if (node1 == nullptr || node2 == nullptr)
return false;
// 如果两个节点值不同,那么它们不可能同构
if (node1->val != node2->val)
return false;
// 检查左右子节点是否也同构
return isIsomorphic(node1->left, node2->left) && isIsomorphic(node1->right, node2->right);
}
// 主函数测试
int main() {
// 创建示例二叉树,例如:
// bt1 = [1, 2, 3]
// / \
// 2 3
// / \
// 3 4
// bt2 = [1, 3, 2]
// /
// 3
// /
// 4
TreeNode* bt1 = new TreeNode(1);
bt1->left = new TreeNode(2);
bt1->right = new TreeNode(3);
bt1->left->left = new TreeNode(3);
bt1->left->right = new TreeNode(4);
TreeNode* bt2 = new TreeNode(1);
bt2->left = new TreeNode(3);
bt2->right = new TreeNode(2);
bt2->left->right = new TreeNode(4);
bool areIsomorphic = isIsomorphic(bt1, bt2);
if (areIsomorphic)
cout << "两棵二叉树同构" << endl;
else
cout << "两棵二叉树非同构" << endl;
delete bt1;
delete bt2; // 释放内存
return 0;
}
```
阅读全文
相关推荐
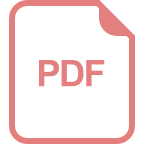
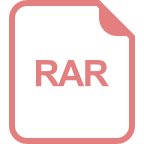
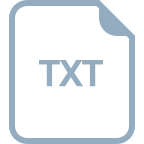















