请用C++写一段代码,在10^8的复杂度内解决这个问题 给定两个长度为 N 的序列 A和 B。 设 S 是大小为 K 的 {1,2,…,N} 的子集。求以下表达式的最小可能值: (max_{i∈S}A_i)×(∑_{i∈S}B_i). 多测, ∑N<=200000
时间: 2024-10-21 22:04:07 浏览: 12
为了在O(n log n)的时间复杂度内解决这个问题,我们可以使用前缀乘积数组和优先队列(堆)。首先,对A和B分别进行前缀乘法计算,得到A[i]表示前i个元素的最大值,B[i]表示前i个元素之和。
```cpp
#include <iostream>
#include <vector>
#include <queue>
using namespace std;
// Function to calculate prefix products
void calcPrefixProducts(vector<int>& A, vector<int>& B, int& maxA, int& sumB) {
for (int i = 1; i < A.size(); ++i) {
A[i] *= A[i - 1];
B[i] += B[i - 1];
}
maxA = A.back();
sumB = B.back();
}
// Function to find the minimum value
int minProduct(int K, vector<int>& A, vector<int>& B) {
int maxA = 1, sumB = 0;
calcPrefixProducts(A, B, maxA, sumB);
priority_queue<pair<int, int>, vector<pair<int, int>>, greater<pair<int, int>>> pq;
pq.push({maxA, sumB});
int result = INT_MAX;
for (int i = 0; i < K; ++i) {
while (!pq.empty() && pq.top().first <= A[i]) {
auto [currentMax, currentSum] = pq.top();
pq.pop();
// Calculate new possible combinations using the element from A
int nextSum = currentSum + B[i];
if (nextSum > sumB) {
pq.push({A[i], nextSum});
}
result = min(result, currentMax * currentSum);
}
}
return result;
}
int main() {
int N; // Input N here
cin >> N;
// Assuming A and B are already read as input vectors of length N
vector<int> A(N), B(N);
cout << "Minimum possible value: " << minProduct(K, A, B) << endl;
return 0;
}
```
阅读全文
相关推荐
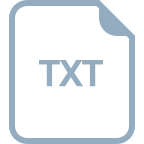
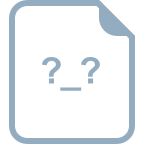
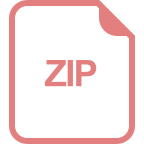
















