写一个递归函数,交换二叉树t中所有结点的左子树和右子树。
时间: 2023-12-08 14:01:44 浏览: 37
递归函数的思路是,首先判断当前节点是否为空,如果为空则直接返回。否则,交换当前节点的左子树和右子树,然后递归地对左子树和右子树进行交换。下面是一个示例的递归函数实现:
```python
def swap_tree(t):
if not t: # 如果当前节点为空,直接返回
return
t.left, t.right = t.right, t.left # 交换当前节点的左右子树
swap_tree(t.left) # 递归对左子树进行交换
swap_tree(t.right) # 递归对右子树进行交换
```
使用该递归函数可以交换二叉树中所有节点的左子树和右子树。在函数中,通过判断当前节点是否为空来结束递归,然后通过交换左右子树的位置来实现交换。最后,再递归地对左子树和右子树进行同样的操作,即不断向下递归交换左右子树。整个过程会遍历每个节点并交换其左右子树,从而实现了交换整个二叉树的左右子树的目的。
相关问题
编写递归算法,将二叉树中所有结点的左右子树相互交换
### 回答1:
递归算法可以通过以下步骤实现二叉树中所有结点的左右子树相互交换:
1. 如果二叉树为空,则返回空。
2. 如果二叉树不为空,则交换当前结点的左右子树。
3. 递归调用交换左子树和右子树。
下面是递归算法的代码实现:
```
void swapTree(TreeNode* root) {
if (root == NULL) {
return;
}
TreeNode* temp = root->left;
root->left = root->right;
root->right = temp;
swapTree(root->left);
swapTree(root->right);
}
```
其中,TreeNode是二叉树结点的定义,包含左右子树指针。在函数中,首先判断当前结点是否为空,如果为空则直接返回。如果不为空,则交换当前结点的左右子树,并递归调用交换左子树和右子树的函数。这样,就可以实现二叉树中所有结点的左右子树相互交换。
### 回答2:
本题要求编写递归算法,将二叉树中所有结点的左右子树相互交换。这是一个非常经典的题目,解决方法就是利用递归算法,先交换当前节点的左右子树,然后递归交换左子树和右子树。
递归算法是一种非常高效的算法,因为它可以将问题分解成许多小问题,然后通过递归的方式解决这些小问题。对于二叉树的递归操作,我们可以先沿着二叉树的左子树一直递归到底,然后再回溯到当前节点的右子树继续递归。
具体实现过程如下:
``` python
def invertTree(root):
if root is None:
return None
# 递归交换左右子树
root.left, root.right = root.right, root.left
# 递归交换左子树和右子树
invertTree(root.left)
invertTree(root.right)
return root
```
递归的停止条件是当前节点为空节点。在递归过程中,我们先交换当前节点的左右子树,然后再递归交换左子树和右子树。最后返回根节点。
这段代码可以实现将二叉树中所有结点的左右子树相互交换的功能,具有很高的实用价值。
### 回答3:
递归实现二叉树的左右子树交换需要考虑以下几个步骤:
1. 当前节点为 null 或叶子节点,则返回该节点
2. 递归处理当前节点的左子树(返回结果为交换后的左子树)
3. 递归处理当前节点的右子树(返回结果为交换后的右子树)
4. 交换当前节点的左右子节点
5. 返回交换后的当前节点
具体实现如下:
```
class Node {
int val;
Node left;
Node right;
Node(int val) {
this.val = val;
this.left = null;
this.right = null;
}
}
public Node swap(Node root) {
// 如果当前节点为 null 或叶子节点,则返回该节点
if (root == null || (root.left == null && root.right == null)) return root;
// 递归处理当前节点的左右子树
Node left = swap(root.left);
Node right = swap(root.right);
// 交换当前节点的左右子节点
root.left = right;
root.right = left;
return root;
}
```
该算法的时间复杂度为 O(n),其中 n 为二叉树的节点数,因为需要遍历每个节点进行交换。空间复杂度为 O(h),其中 h 为二叉树的高度,因为需要递归调用栈来保存调用信息。
编写递归算法,将二叉树中所有结点的左右子树相互交换。
递归地交换二叉树节点的左右子树,可以使用下面的算法:
1. 如果二叉树为空,返回 NULL。
2. 如果二叉树非空,则交换左右子树。
3. 递归交换左子树。
4. 递归交换右子树。
5. 返回交换后的根节点。
下面是实现这个算法的递归函数:
```python
def invert_tree(root):
if not root:
return None
root.left, root.right = root.right, root.left
invert_tree(root.left)
invert_tree(root.right)
return root
```
该函数接受一个二叉树的根节点作为参数,并返回交换后的根节点。如果根节点为空,函数返回 None。否则,函数首先交换根节点的左右子树,然后递归地交换左子树和右子树,并返回交换后的根节点。
相关推荐
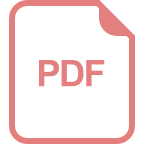












