请用C++语言编程,先使用进退法搜索单峰区间,再进而使用黄金分割法计算函数为f ( x ) = e 的(− x)次方 + x 平方 − 2 x的最小值
时间: 2024-12-22 21:18:00 浏览: 6
在C++中,我们可以采用二分查找的进阶方法,如牛顿法或者黄金分割法来寻找单峰区间的最小值。首先,我们需要使用二分搜索找到一个近似的单峰区间,然后利用黄金分割法进一步精确地定位最小值。以下是简化的示例代码:
```cpp
#include <iostream>
#include <cmath>
#include <vector>
// 进退法寻找单峰区间
std::pair<double, double> binary_search_f(double(*func)(double), double a, double b) {
while (a <= b) {
double c = (a + b) / 2;
if (func(c) * func(a) > 0) // 如果左半边递增,右半边递减
b = c; // 更新右边界
else
a = c + 1; // 否则更新左边界
}
return {a, b}; // 返回单峰区间的左右端点
}
// 黄金分割法优化搜索
double golden_section_search_f(double(*func)(double), double left, double right) {
double golden_ratio = (sqrt(5) - 1) / 2;
for (int i = 0; i < 5; ++i) {
double c = left + golden_ratio * (right - left);
double d = left + 1 - golden_ratio * (right - left);
if (func(d) < func(c))
right = c;
else
left = d;
}
return func(left); // 返回最接近最小值的位置对应的函数值
}
// 函数 f(x)
double func(double x) {
return std::exp(-x) + x * x - 2 * x;
}
int main() {
double left, right;
std::tie(left, right) = binary_search_f(func, -10, 10); // 确定初始的单峰区间
double min_val = golden_section_search_f(func, left, right); // 使用黄金分割法找出最小值
std::cout << "The minimum value of the function is: " << min_val << std::endl;
return 0;
}
```
阅读全文
相关推荐
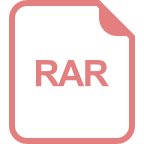
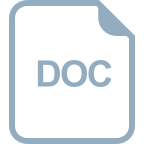
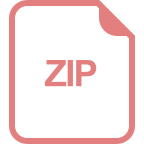





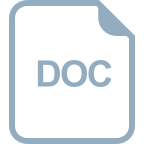
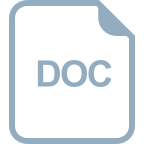
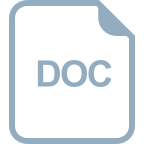
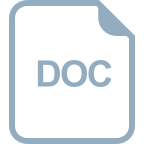
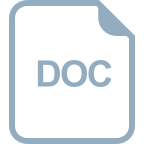





