nn.Conv2d hidden_dim
时间: 2023-11-23 08:55:48 浏览: 168
根据提供的引用内容,nn.Conv2d方法是PyTorch中用于实现二维卷积的方法。其中,hidden_dim是指卷积层的输出通道数,即卷积核的数量。在nn.Conv2d方法中,可以通过设置参数out_channels来指定hidden_dim的值。下面是一个示例代码:
```python
import torch.nn as nn
# 定义一个卷积层,输入通道数为3,输出通道数为16,卷积核大小为3x3
conv = nn.Conv2d(in_channels=3, out_channels=16, kernel_size=3)
# 打印卷积层的参数
print(conv)
# 输出:
# Conv2d(3, 16, kernel_size=(3,3), stride=(1, 1))
```
在上面的代码中,我们定义了一个输入通道数为3,输出通道数为16,卷积核大小为3x3的卷积层。可以看到,输出的卷积层参数中,out_channels的值为16,即hidden_dim为16。
相关问题
class GhostModule(nn.Module): def __init__(self, input_channels, output_channels, kernel_size=1, ratio=2): super(GhostModule, self).__init__() self.output_channels = output_channels self.hidden_channels = output_channels // ratio self.primary_conv = nn.Sequential( nn.Conv2d(input_channels, self.hidden_channels, kernel_size, bias=False), nn.BatchNorm2d(self.hidden_channels), nn.ReLU(inplace=True) ) self.cheap_operation = nn.Sequential( nn.Conv2d(self.hidden_channels, self.hidden_channels, kernel_size, groups=self.hidden_channels, bias=False), nn.BatchNorm2d(self.hidden_channels), nn.ReLU(inplace=True) ) self.secondary_conv = nn.Sequential( nn.Conv2d(self.hidden_channels, self.output_channels - self.hidden_channels, kernel_size, bias=False), nn.BatchNorm2d(self.output_channels - self.hidden_channels) ) def forward(self, x): x1 = self.primary_conv(x) x2 = self.cheap_operation(x1) x3 = self.secondary_conv(torch.cat([x1, x2], dim=1)) return torch.cat([x1, x3], dim=1)
这是一个 PyTorch 的神经网络模块,该模块名为 GhostModule。该模块包含三个卷积层:主要卷积层、廉价卷积操作和次要卷积层。其中主要卷积层是一个标准的卷积操作,廉价卷积操作是一种深度可分离卷积,次要卷积层是一个标准的卷积操作。这个模块的目的是在减少计算成本的同时保持模型的准确性。你有没有其他问题呢?
import torch.nn as nn from torch.nn import functional as F class InvertedResidual(nn.Module): def __init__(self, in_features, out_features, stride, expand_ratio,activation=nn.ReLU6) : super(InvertedResidual, self).__init__() self.stride = stride assert stride in [1, 2] hidden_dim = in_features * expand_ratio self.is_residual = self.stride == 1 and in_features == out_features self.conv = nn.Sequential( # pw Point-wise nn.Conv2d(in_features, hidden_dim, 1, 1, 0, bias=False), nn.BatchNorm2d(hidden_dim), activation(inplace=True), # dw Depth-wise nn.Conv2d(hidden_dim, hidden_dim, 3, stride, 1, groups=hidden_dim, bias=False), nn.BatchNorm2d(hidden_dim), activation(inplace=True), # pw-linear, Point-wise linear nn.Conv2d(hidden_dim, out_features, 1, 1, 0, bias=False), nn.BatchNorm2d(out_features), ) def forward(self, x): if self.is_residual: return x + self.conv(x) else: return self.conv(x) print(InvertedResidual(1280, 512, stride=2, expand_ratio=6) )
这段代码定义了一个名为InvertedResidual的类,继承自nn.Module。该类包含了一些参数,如输入特征、输出特征、步长、扩张比例和激活函数。在类的初始化函数中,会根据这些参数创建一个卷积神经网络模型,并且判断是否需要进行残差连接。其中,nn.ReLU6是一个激活函数,nn.Sequential是一个容器,可以将多个层组合在一起。
阅读全文
相关推荐
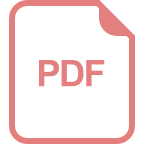
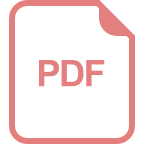
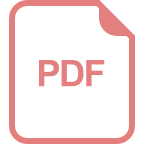













