用python pytochj写一个 5帧数据输入 包含 卷积+convLSTM 卷积操作块+conv+BatchNorm2d+分割输出
时间: 2024-01-11 07:05:36 浏览: 69
好的,这个问题是技术问题,可以回答。以下是一个示例代码:
```python
import torch
import torch.nn as nn
class ConvLSTMCell(nn.Module):
def __init__(self, input_size, hidden_size):
super(ConvLSTMCell, self).__init__()
self.input_size = input_size
self.hidden_size = hidden_size
self.Gates = nn.Conv2d(input_size + hidden_size, 4 * hidden_size, 3, padding = 1)
def forward(self, input, hidden, cell):
combined = torch.cat((input, hidden), dim=1)
gates = self.Gates(combined)
forget_gate, input_gate, output_gate, cell_gate = gates.chunk(4, dim=1)
forget_gate = torch.sigmoid(forget_gate)
input_gate = torch.sigmoid(input_gate)
cell_gate = torch.tanh(cell_gate)
output_gate = torch.sigmoid(output_gate)
cell = (forget_gate * cell) + (input_gate * cell_gate)
hidden = output_gate * torch.tanh(cell)
return hidden, cell
class ConvLSTMLayer(nn.Module):
def __init__(self, input_size, hidden_size):
super(ConvLSTMLayer, self).__init__()
self.input_size = input_size
self.hidden_size = hidden_size
self.ConvLSTMCell = ConvLSTMCell(input_size, hidden_size)
def forward(self, input, Hidden_State=None, Cell_State=None):
if Hidden_State is None:
Hidden_State = torch.zeros(input.size()[0], self.hidden_size, input.size()[2], input.size()[3]).cuda()
if Cell_State is None:
Cell_State = torch.zeros(input.size()[0], self.hidden_size, input.size()[2], input.size()[3]).cuda()
Hidden_State_List = []
for timestep in range(input.size()[1]):
Hidden_State, Cell_State = self.ConvLSTMCell(input[:,timestep,:,:,:], Hidden_State, Cell_State)
Hidden_State_List.append(Hidden_State)
return torch.stack(Hidden_State_List, dim=1), Hidden_State, Cell_State
class ConvBlock(nn.Module):
def __init__(self, in_channels, out_channels):
super(ConvBlock, self).__init__()
self.conv = nn.Sequential(
nn.Conv2d(in_channels, out_channels, kernel_size=3, stride=1, padding=1, bias=False),
nn.BatchNorm2d(out_channels),
nn.ReLU(inplace=True)
)
def forward(self, x):
return self.conv(x)
class SegmentationModel(nn.Module):
def __init__(self):
super(SegmentationModel, self).__init__()
self.convlstm_layer = ConvLSTMLayer(512, 512)
self.conv_block1 = ConvBlock(512, 256)
self.conv_block2 = ConvBlock(256, 128)
self.classification = nn.Sequential(
nn.Conv2d(128, 2, kernel_size=1, stride=1, padding=0),
nn.BatchNorm2d(2),
nn.Softmax(dim=1)
)
def forward(self, x):
x, _, _ = self.convlstm_layer(x)
x = self.conv_block1(x)
x = self.conv_block2(x)
x = self.classification(x)
return x
```
这是一个简单的分割模型,由ConvLSTMLayer和两个ConvBlock组成,输入5帧大小为512 x W x H的数据,输出大小为2 x W x H的分割结果。其中ConvLSTMLayer是一个5帧ConvLSTM层,用于学习时序信息。ConvBlock是一个包含卷积和BatchNorm的卷积操作块,用于提取特征。分类层使用1x1卷积进行2分类,并使用Softmax进行归一化。
阅读全文
相关推荐
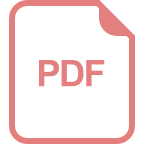
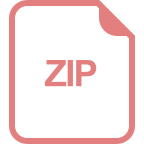
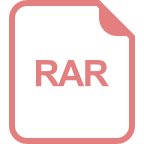















