作业调度问题优先队列分支限界
时间: 2025-01-05 08:36:00 浏览: 11
### 使用优先队列实现分支限界法解决作业调度问题
#### 1. 背景介绍
批处理作业调度问题是经典的组合优化问题之一,目标是从多个可能的作业序列中找到使总完成时间最短的一个。当涉及多台机器时,该问题变得更加复杂。为了有效地求解此类问题,可以采用分支限界法并结合优先队列来加速搜索过程。
#### 2. 方法概述
通过构建一颗排列树表示所有可能的任务分配方式,并利用界限函数剪枝不必要的子节点探索路径。在此基础上引入优先队列管理待考察的状态节点,按照估计成本从小到大顺序选取下一个扩展结点,从而提高算法效率[^1]。
#### 3. 关键概念解释
- **状态空间树**:用于描述不同阶段下各个任务被安排的情况;
- **活结点列表 (EPL)** :存储当前未完全展开但仍有可能成为最优解的部分解决方案集合;
- **上界计算方法**:基于已知条件预测剩余部分最低消耗的时间开销作为评价标准;
#### 4. 算法流程说明
初始化时将根节点加入到优先队列中,随后重复执行以下操作直到获得满意的结果:
- 取出队首元素即具有最小预计代价的活动节点;
- 如果此节点代表完整的可行解,则记录下来并与现有最好结果比较更新全局最优值;
- 否则对该节点进行进一步划分生成新的候选方案,并根据预估得分重新排序入队等待后续评估。
#### 5. Python代码示例
下面给出一段简单的Python伪代码展示如何应用上述思路解决问题:
```python
import heapq
def branch_and_bound(n, processing_times):
class Node:
def __init__(self, level, path, cost, bound):
self.level = level # 当前层数/深度
self.path = list(path) # 已经决定的工作次序
self.cost = cost # 到达此处累积花费
self.bound = bound # 预测最终所需最少时间
def __lt__(self, other):
return self.bound < other.bound
min_cost = float('inf')
best_solution = None
start_node = Node(0, [], 0, calculate_bound([], n))
live_nodes = []
heapq.heappush(live_nodes, start_node)
while len(live_nodes)>0:
current = heapq.heappop(live_nodes)
if current.level == n:
if current.cost < min_cost:
min_cost = current.cost
best_solution = current.path
elif current.bound < min_cost:
for i in range(len(processing_times)):
if not visited[i]:
new_path = current.path + [i]
next_level = current.level + 1
temp_visited = visited.copy()
temp_visited[i]=True
accumulated_time = sum([processing_times[new_path[j]][j] for j in range(next_level)])
estimated_remaining = estimate_lower_bound(new_path, remaining_jobs(temp_visited), n)
child = Node(
next_level,
new_path,
accumulated_time,
accumulated_time + estimated_remaining
)
heapq.heappush(live_nodes,child)
return best_solution, min_cost
def calculate_bound(partial_sequence, total_tasks):
""" 计算给定部分序列下的边界 """
pass
def estimate_lower_bound(current_ordering, rest_of_jobs, num_machines):
""" 对于剩下的工作估算其最早结束时刻"""
pass
def remaining_jobs(is_selected):
""" 获取尚未选择的任务索引列表"""
pass
```
阅读全文
相关推荐
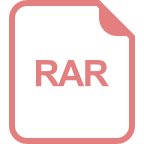

















