编写一个程序来解决给定的迷宫问题。迷宫由一个二维数组表示,0。表示通道,1。表示墙壁,起点和终点分别由坐标给出。程序需要找到起点到终点的最短路径,并输出路径。
时间: 2024-09-10 09:28:07 浏览: 126
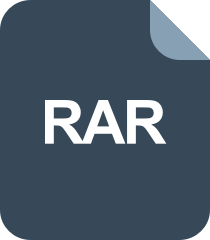
migong.rar_迷宫问题 程序设计
编写一个程序来解决迷宫问题可以通过多种算法实现,其中较为常用的是深度优先搜索(DFS)和广度优先搜索(BFS)。这里以BFS为例,因为它能够更自然地找到最短路径。以下是使用BFS算法求解迷宫问题的基本步骤:
1. 将起点加入队列中,并将起点位置标记为已访问。
2. 当队列不为空时,循环执行以下步骤:
a. 从队列中取出一个位置(当前位置)。
b. 如果当前位置是终点,结束搜索。
c. 否则,查看当前位置的四个方向(上、下、左、右)的邻居。
d. 对于每一个未访问过的邻居,如果它不是墙壁(即值为0),将其加入队列,并标记为已访问,同时记录它的前驱位置,以便后续回溯路径。
3. 搜索结束后,根据记录的前驱位置回溯,从终点开始,逐个前驱地找到起点,这就是最短路径。
下面是一个简单的Python代码示例,演示了如何实现上述算法:
```python
from collections import deque
def find_shortest_path(maze, start, end):
rows, cols = len(maze), len(maze[0])
visited = [[False for _ in range(cols)] for _ in range(rows)]
prev = [[None for _ in range(cols)] for _ in range(rows)]
directions = [(0, 1), (1, 0), (0, -1), (-1, 0)]
queue = deque([start])
visited[start[0]][start[1]] = True
while queue:
x, y = queue.popleft()
if (x, y) == end:
return reconstruct_path(prev, start, end)
for dx, dy in directions:
nx, ny = x + dx, y + dy
if 0 <= nx < rows and 0 <= ny < cols and not visited[nx][ny] and maze[nx][ny] == 0:
queue.append((nx, ny))
visited[nx][ny] = True
prev[nx][ny] = (x, y)
return None
def reconstruct_path(prev, start, end):
path = [end]
while prev[end[0]][end[1]] is not None:
end = prev[end[0]][end[1]]
path.append(end)
path.reverse()
return path if path[0] == start else None
# 测试代码
maze = [
[0, 1, 0, 0, 0],
[0, 1, 0, 1, 0],
[0, 0, 0, 1, 0],
[0, 1, 1, 1, 0],
[0, 0, 0, 0, 0]
]
start = (0, 0)
end = (4, 4)
path = find_shortest_path(maze, start, end)
print("最短路径:", path)
```
在这个示例中,我们首先定义了一个`find_shortest_path`函数,它接受迷宫、起点和终点作为参数,并使用BFS算法来找到最短路径。然后,我们定义了一个`reconstruct_path`函数,用于根据前驱位置记录回溯整个路径。最后,我们使用了一个简单的迷宫、起点和终点来测试这个算法,并打印出了最短路径。
阅读全文
相关推荐
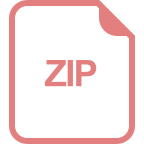
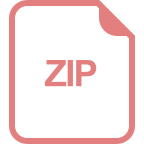
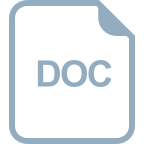
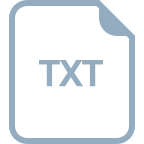
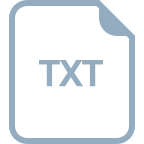
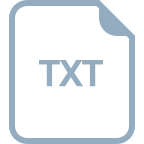
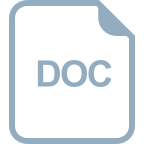
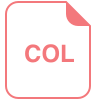









