java开心消消乐代码
时间: 2023-07-02 10:07:38 浏览: 203
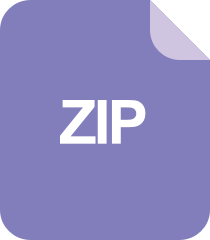
html开心消消乐源码

以下是一个简单的Java代码实现开心消消乐游戏:
```java
import java.util.*;
public class HappyElimination {
private static final int ROWS = 8; // 行数
private static final int COLS = 8; // 列数
private static final int COLORS = 5; // 颜色数
private static final int[][] NEIGHBORS = {{-1, 0}, {1, 0}, {0, -1}, {0, 1}}; // 上下左右四个方向
private static int[][] grid = new int[ROWS][COLS]; // 网格
public static void main(String[] args) {
generateGrid(); // 生成网格
printGrid(); // 打印网格
int moves = 0; // 移动次数
while (true) {
Set<Point> toRemove = findMatches(); // 查找并记录所有可消除的点
if (toRemove.isEmpty()) { // 如果没有点可以消除,结束游戏
System.out.println("Game over!");
break;
}
removePoints(toRemove); // 消除所有可消除的点
collapseGrid(); // 让所有点下落
fillGrid(); // 填充新的随机点
moves++; // 增加移动次数
printGrid(); // 打印网格
}
System.out.println("Total moves: " + moves);
}
// 生成随机网格
private static void generateGrid() {
Random random = new Random();
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
grid[i][j] = random.nextInt(COLORS) + 1;
}
}
}
// 打印网格
private static void printGrid() {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
System.out.print(grid[i][j] + " ");
}
System.out.println();
}
System.out.println();
}
// 查找所有可消除的点
private static Set<Point> findMatches() {
Set<Point> toRemove = new HashSet<>();
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
int color = grid[i][j];
if (color == 0) {
continue; // 已经被标记为待消除的点
}
Set<Point> matches = new HashSet<>();
findMatchesHelper(i, j, color, matches); // 查找相邻颜色相同的点
if (matches.size() >= 3) { // 如果有3个或以上相邻颜色相同的点,记录下来
toRemove.addAll(matches);
}
}
}
return toRemove;
}
// 递归查找相邻颜色相同的点
private static void findMatchesHelper(int row, int col, int color, Set<Point> matches) {
if (row < 0 || row >= ROWS || col < 0 || col >= COLS || grid[row][col] != color) {
return;
}
Point p = new Point(row, col);
if (matches.contains(p)) {
return;
}
matches.add(p);
for (int[] neighbor : NEIGHBORS) {
findMatchesHelper(row + neighbor[0], col + neighbor[1], color, matches);
}
}
// 消除所有可消除的点
private static void removePoints(Set<Point> toRemove) {
for (Point p : toRemove) {
grid[p.row][p.col] = 0; // 标记为待消除的点
}
}
// 让所有点下落
private static void collapseGrid() {
for (int col = 0; col < COLS; col++) {
int row = ROWS - 1;
while (row >= 0) {
if (grid[row][col] == 0) { // 如果是待消除的点,跳过
row--;
} else if (row == ROWS - 1) { // 如果是最底部的点,不用做任何事情
row--;
} else { // 向下移动点
int temp = grid[row][col];
grid[row][col] = 0;
int newRow = row + 1;
while (newRow < ROWS && grid[newRow][col] == 0) {
newRow++;
}
grid[newRow - 1][col] = temp;
row--;
}
}
}
}
// 填充新的随机点
private static void fillGrid() {
Random random = new Random();
for (int col = 0; col < COLS; col++) {
if (grid[0][col] == 0) { // 如果顶部是空的,填充新的随机点
for (int row = 0; row < ROWS; row++) {
if (grid[row][col] != 0) {
break;
}
grid[row][col] = random.nextInt(COLORS) + 1;
}
}
}
}
// 表示一个点的坐标
private static class Point {
int row;
int col;
Point(int row, int col) {
this.row = row;
this.col = col;
}
@Override
public int hashCode() {
return Objects.hash(row, col);
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null || getClass() != obj.getClass()) {
return false;
}
Point other = (Point) obj;
return row == other.row && col == other.col;
}
}
}
```
代码中使用一个二维数组来表示游戏网格,每个元素表示一个点的颜色。游戏的主要逻辑在 `main()` 方法中实现,通过不断查找可消除的点,消除所有可消除的点,让所有点下落,填充新的随机点,直到没有可消除的点为止,算出总的移动次数并输出。
阅读全文
相关推荐

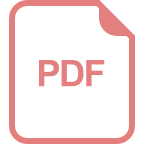



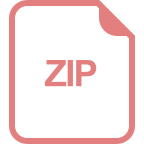
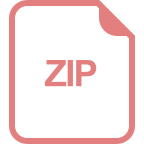
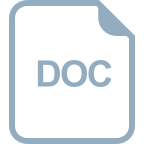
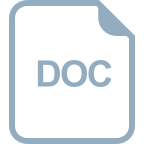







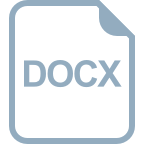