class ResNet(nn.Module): def __init__(self, num_classes=32): super(ResNet, self).__init__() self.conv1 = nn.Conv2d(3, 64, kernel_size=7, stride=2, padding=3) self.bn1 = nn.BatchNorm2d(64) self.relu = nn.ReLU(inplace=True) self.maxpool = nn.MaxPool2d(kernel_size=3, stride=2, padding=1) self.layer1 = nn.Sequential( ResNetBlock(64, 64), ResNetBlock(64, 64), ResNetBlock(64, 64) ) self.layer2 = nn.Sequential( ResNetBlock(64, 128), ResNetBlock(128, 128), ResNetBlock(128, 128) ) self.layer3 = nn.Sequential( ResNetBlock(128, 256), ResNetBlock(256, 256), ResNetBlock(256, 256) ) self.layer4 = nn.Sequential( ResNetBlock(256, 512), ResNetBlock(512, 512), ResNetBlock(512, 512) ) self.avgpool = nn.AdaptiveAvgPool2d((1, 1)) self.fc = nn.Linear(512, num_classes) def forward(self, x): x = self.conv1(x) x = self.bn1(x) x = self.relu(x) x = self.maxpool(x) x = self.layer1(x) x = self.layer2(x) x = self.layer3(x) x = self.layer4(x) x = self.avgpool(x) x = x.view(x.size(0), -1) x = self.fc(x) return x 解释该代码
时间: 2023-06-13 22:08:21 浏览: 151
这段代码定义了一个 ResNet 模型,用于图像分类任务,包括以下几个部分:
1. 初始化函数 `__init__` 中,定义了模型的各个层,包括了卷积层、Batch Normalization 层、ReLU 激活函数、最大池化层、ResNetBlock 残差块、自适应平均池化层和全连接层。
2. `forward` 函数中,定义了模型的前向传播过程,将输入的图像数据经过一系列的卷积、池化和残差块的操作后,经过自适应平均池化层将特征图变成一个固定大小的向量,最后通过全连接层映射成分类结果。
3. 该模型使用了 ResNetBlock 残差块,用于解决深度网络中的梯度消失问题,每个残差块中包含了两个卷积层和一个跳跃连接,保证了信息的流动和梯度的稳定。
相关问题
class ResNet_101(nn.Module): def __init__(self, num_classes): super(ResNet_101, self).__init__() model = torchvision.models.resnet50(pretrained=True) model.fc = nn.Sequential( nn.Linear(model.fc.in_features, num_classes, bias=True), ) self.net = model def forward(self, img): output = self.net(img) return output如何做def state_dict(self):
你可以使用`state_dict()`方法来返回当前模型的状态字典。状态字典是一个Python字典对象,其中包含模型的所有参数。在这个示例中,你可以通过以下方式实现`state_dict()`方法:
```python
def state_dict(self):
state_dict = {
'model': self.net.state_dict(),
}
return state_dict
```
在这个方法中,你创建了一个名为`state_dict`的字典对象,并将整个模型的状态字典存储在其中的一个键值对中,键为`'model'`。你可以使用`self.net.state_dict()`方法来获取`ResNet_101`模型内部的所有参数的状态字典。最后,你返回整个状态字典对象。
class ResNetRGBD(nn.Module): def __init__(self, block, layers, num_classes=1000, zero_init_residual=False, groups=1, width_per_group=64, replace_stride_with_dilation=None, norm_layer=None): super(ResNetRGBD, self).__init__() if norm_layer is None: norm_layer = nn.BatchNorm2d self._norm_layer = norm_layer self.inplanes = 64 self.dilation = 1
这是一个 PyTorch 中的 ResNetRGBD 模型类的定义,它继承自 nn.Module 类。在初始化函数 `__init__` 中,它接受以下参数:
- `block`:ResNet 中残差块的类(比如 BasicBlock 或 Bottleneck)。
- `layers`:一个列表,表示每个残差块堆叠的数量。
- `num_classes`:输出类别的数量。
- `zero_init_residual`:是否使用零初始化残差块的权重。
- `groups`:分组卷积中的组数。
- `width_per_group`:每个组的通道数。
- `replace_stride_with_dilation`:是否使用空洞卷积代替步幅卷积来降低下采样率。
- `norm_layer`:归一化层的类型(比如 BatchNorm2d 或 GroupNorm)。
其中,`self._norm_layer` 表示使用的归一化层,`self.inplanes` 表示当前的通道数,`self.dilation` 表示当前的空洞率。
阅读全文
相关推荐
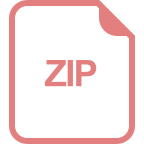
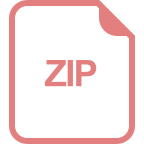
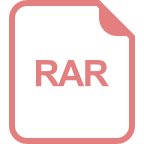













