基于stm32f103使用红外传感器避障代码
时间: 2024-01-24 15:03:44 浏览: 34
以下是一个基于STM32F103芯片的红外避障程序代码,使用红外传感器模块检测障碍物并控制车辆避开障碍物。
```c
#include "stm32f10x.h"
#include "delay.h"
#include "usart.h"
#define LedPB5 GPIO_Pin_5
#define LedPB5_ON() GPIO_ResetBits(GPIOB,LedPB5)
#define LedPB5_OFF() GPIO_SetBits(GPIOB,LedPB5)
#define InfraredPA0 GPIO_Pin_0
#define InfraredPA0_ON() GPIO_ResetBits(GPIOA,InfraredPA0)
#define InfraredPA0_OFF() GPIO_SetBits(GPIOA,InfraredPA0)
int main(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB | RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Pin = LedPB5;
GPIO_Init(GPIOB, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_InitStructure.GPIO_Pin = InfraredPA0;
GPIO_Init(GPIOA, &GPIO_InitStructure);
USART1_Configuration();
while(1)
{
if(GPIO_ReadInputDataBit(GPIOA,InfraredPA0) == RESET)
{
printf("Infrared obstacle detected!\r\n");
LedPB5_ON();
//turn left
//...
DelayMs(500); //delay 500ms
LedPB5_OFF();
}
else
{
printf("No obstacle found.\r\n");
LedPB5_OFF();
//move forward
//...
}
}
}
```
在这个程序中,我们首先初始化了STM32的GPIO引脚,将LedPB5引脚配置为输出模式,InfraredPA0引脚配置为上拉输入模式。然后我们进入了一个无限循环,在循环中我们检测红外传感器是否检测到障碍物。如果检测到障碍物,我们就输出一条提示信息,并且点亮LedPB5引脚表示有障碍物。此时可以控制车辆避开障碍物,比如向左转。如果没有检测到障碍物,我们就输出另一条提示信息,并且熄灭LedPB5引脚表示没有障碍物,此时可以继续向前行驶。
需要注意的是,在实际使用中,需要根据具体的硬件设备和实际情况来修改程序,比如修改引脚号、修改延时时间、控制车辆的具体行动等。
相关推荐
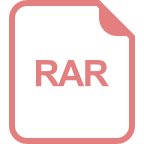
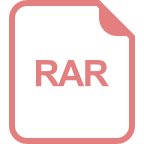














