import pygame import g29_controller pygame.init() BLACK = (0, 0, 0) WHITE = (255, 255, 255) RED = (255, 0, 0) GREEN = (0, 255, 0) BLUE = (0, 0, 255) windowSize = (900, 600) window = pygame.display.set_mode(windowSize) pygame.display.set_caption("G29 Controller") FPS = 10 clock = pygame.time.Clock() done = False controller = g29_controller.Controller(0) while not done: for event in pygame.event.get(): if event.type == pygame.QUIT: done = True # handle joysticks jsButtons = controller.get_buttons() jsInputs = controller.get_axis() steerPos = controller.get_steer() throtPos = controller.get_throttle() breakPos = controller.get_break() clutchPos = controller.get_clutch() steerV = bytes([128 + int(steerPos * 128)]) throtV = bytes([128 + int(throtPos * 128)]) breakV = bytes([128 + int(breakPos * 128)]) clutchV = bytes([128 + int(clutchPos * 128)]) if steerPos >= 0: ball_color = RED else: ball_color = GREEN window.fill(BLACK) plh = [] btn = [] axis = [] # axisPlh = [] axis.append(int.from_bytes(steerV)) axis.append(int.from_bytes(throtV)) axis.append(int.from_bytes(breakV)) axis.append(int.from_bytes(clutchV)) for i in range(len(jsButtons)): plh.append("%d") btn.append(jsButtons[i]) # if i < 5: axisPlh.append("%d") font = pygame.font.Font('freesansbold.ttf', 32) ph = " ".join(plh) aph = " ".join(plh[:4]) btn = tuple(btn) btnText = font.render(ph % btn, True, WHITE) axisText = font.render(aph % tuple(axis), True, WHITE) btnTextRect = btnText.get_rect() axisTextRect = axisText.get_rect() btnTextRect.center = (450, 300) axisTextRect.center = (450, 400) window.blit(btnText, btnTextRect) window.blit(axisText, axisTextRect) pygame.display.flip() clock.tick(FPS) # quit app. pygame.quit()
时间: 2024-04-28 19:21:49 浏览: 13
这是一个使用pygame和g29_controller模块编写的Python程序。程序实现了对G29游戏方向盘的控制和读取。在程序中,通过Controller类的方法获取游戏方向盘的各种状态信息,并将这些信息显示在Pygame窗口中。其中,get_buttons()方法用于获取游戏方向盘的按钮状态,get_axis()方法用于获取游戏方向盘的各轴状态,get_steer()、get_throttle()、get_break()和get_clutch()方法则用于获取游戏方向盘的转向角度、油门、刹车和离合器状态。程序的while循环不断读取这些状态信息,并将它们转换成对应的字节序列,最后将字节序列渲染成文本显示在Pygame窗口中。
相关问题
详细解释以下代码color_red = pygame.Color(255, 0, 0) color_white = pygame.Color(255, 255, 255) color_green = pygame.Color(0, 255, 0) pygame.init() screen = pygame.display.set_mode((600, 400)) screen.fill(color_white) pygame.display.set_caption("贪吃蛇小游戏") arr = [([0
这段代码是一个使用 Pygame 模块创建一个贪吃蛇小游戏的基本框架。下面是对代码的详细解释:
- 第一行定义了一个名为 color_red 的变量,其值为一个 RGB 颜色值,即 (255, 0, 0),表示红色。
- 第二行定义了一个名为 color_white 的变量,其值为另一个 RGB 颜色值,即 (255, 255, 255),表示白色。
- 第三行定义了一个名为 color_green 的变量,其值为另一个 RGB 颜色值,即 (0, 255, 0),表示绿色。
- 第四行调用 pygame 模块的 init() 函数,用于初始化 pygame 库。
- 第五行调用 pygame 模块的 display.set_mode() 函数,创建一个 600x400 的游戏窗口,并将其赋值给 screen 变量。
- 第六行调用 screen 对象的 fill() 方法,将窗口填充为白色。
- 第七行调用 pygame 模块的 display.set_caption() 函数,设置游戏窗口的标题为“贪吃蛇小游戏”。
- 第八行创建了一个二维列表 arr,用于存储贪吃蛇的位置信息和食物的位置信息。
- 第九行开始了一个 while 循环,该循环将持续运行游戏,直到用户关闭游戏窗口。
- 第十行是一个事件循环,用于检测用户的操作,例如按键、鼠标点击等。
- 第十一行判断如果用户点击了关闭按钮,则退出游戏循环。
- 第十二行通过调用 screen 对象的 blit() 方法,将 arr 列表中的位置信息绘制到游戏窗口上。
- 第十三行调用 pygame 模块的 display.update() 函数,刷新游戏窗口,使得最新的绘制内容显示出来。
import pygame,time pygame.init() screen = pygame.display.set_mode([900, 700]) pygame.display.est_caption(弹球) red = 255,0,0 black = 0,0,0 x = 10 y = 10 dx = 2 dy = 1 redius = 10 KeepGoing = True whil
e KeepGoing:
for event in pygame.event.get():
if event.type == pygame.QUIT:
KeepGoing = False
screen.fill(black)
pygame.draw.circle(screen, red, (x, y), redius)
pygame.display.flip()
x += dx
y += dy
if x < 0 or x > 900:
dx = -dx
if y < 0 or y > 700:
dy = -dy
time.sleep(0.01)
pygame.quit()
这段代码使用pygame库创建了一个窗口,并在窗口中绘制了一个移动的红色球体。程序会不断更新球体的位置,并在窗口中显示。当点击窗口关闭按钮时,程序会退出。
相关推荐
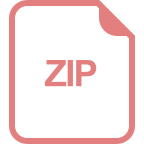
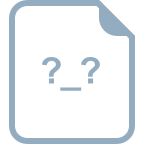












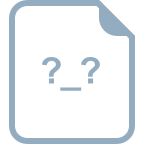