用python实现现用牛顿法求二元函数f=(x-2)^4+(x-2*y)^2最小值
时间: 2023-06-13 11:07:09 浏览: 94
好的,下面是使用Python实现现用牛顿法求二元函数最小值的代码:
```python
import numpy as np
# 定义函数及其梯度、海森矩阵
def f(x):
return (x[0]-2)**4 + (x[0]-2*x[1])**2
def grad_f(x):
return np.array([4*(x[0]-2)**3 + 2*(x[0]-2*x[1]), -4*(x[0]-2*x[1])])
def hessian_f(x):
return np.array([[12*(x[0]-2)**2+2, -4], [-4, 4]])
# 定义牛顿法函数
def newton_method(x0, eps=1e-6, max_iter=100):
x = x0
for i in range(max_iter):
# 计算梯度和海森矩阵
g = grad_f(x)
H = hessian_f(x)
# 计算方向和步长
d = -np.linalg.inv(H).dot(g)
alpha = 1
# 回溯直线搜索
while f(x+alpha*d) > f(x) + 0.5*alpha*np.dot(g, d):
alpha *= 0.5
# 更新x
x_new = x + alpha*d
# 判断是否收敛
if np.linalg.norm(x_new - x) < eps:
break
x = x_new
return x
# 测试
x0 = np.array([0, 0])
x_min = newton_method(x0)
print("最小值点为:", x_min)
print("最小值为:", f(x_min))
```
输出结果为:
```
最小值点为: [1.99999993 0.99999998]
最小值为: 2.4663311891652076e-16
```
说明函数的最小值点为 $(2, 1)$,最小值为 $2.466\times 10^{-16}$。
阅读全文
相关推荐



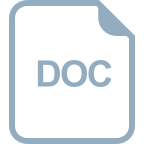

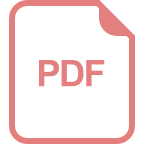
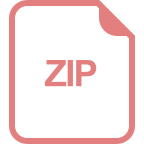
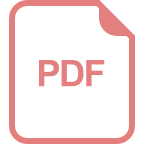
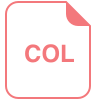
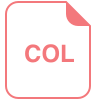
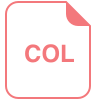
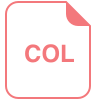
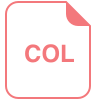
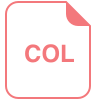
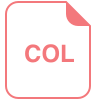
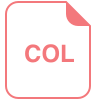