hadoop编写矩阵相乘
时间: 2023-11-14 11:18:29 浏览: 83
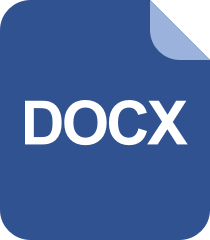
Hadoop矩阵相乘完整实现
在Hadoop中,矩阵相乘可以使用MapReduce来实现。以下是一种可能的实现方式:
1. Mapper阶段:将相乘的两个矩阵中的每个元素都分别作为key-value对传递给Mapper。其中,key为矩阵中的行号,value为该行中的所有元素。
2. Reducer阶段:对于每个key,Reducer会将该key对应的两个矩阵中的行向量分别取出,进行相乘并求和,最终得到结果矩阵中的一个元素。将该元素作为key-value对输出,其中key为结果矩阵中的行号,value为该行中的元素。
下面是一个简单的MapReduce程序:
Mapper代码:
```
public static class MatrixMapper extends Mapper<LongWritable, Text, IntWritable, Text> {
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
// 读取一行数据
String line = value.toString();
String[] tokens = line.split(",");
// 判断是矩阵A还是矩阵B
if (tokens[0].equals("A")) {
// 将元素作为value输出,key为行号
for (int i = 0; i < tokens.length - 2; i++) {
context.write(new IntWritable(Integer.parseInt(tokens[1])), new Text(tokens[i + 2] + ",A," + tokens[i + 1]));
}
} else {
// 将元素作为value输出,key为列号
for (int i = 0; i < tokens.length - 2; i++) {
context.write(new IntWritable(Integer.parseInt(tokens[i + 1])), new Text(tokens[2] + ",B," + tokens[i + 2]));
}
}
}
}
```
Reducer代码:
```
public static class MatrixReducer extends Reducer<IntWritable, Text, Text, Text> {
public void reduce(IntWritable key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
// 两个矩阵的行向量
List<Double> vectorA = new ArrayList<>();
List<Double> vectorB = new ArrayList<>();
// 遍历所有value
for (Text value : values) {
String[] tokens = value.toString().split(",");
if (tokens[1].equals("A")) {
vectorA.add(Double.parseDouble(tokens[0]));
} else {
vectorB.add(Double.parseDouble(tokens[0]));
}
}
// 计算两个向量的点积
double result = 0;
for (int i = 0; i < vectorA.size(); i++) {
result += vectorA.get(i) * vectorB.get(i);
}
// 输出结果
context.write(new Text(key.toString()), new Text(Double.toString(result)));
}
}
```
在驱动程序中,需要设置输入输出路径,以及Mapper和Reducer类:
```
Job job = Job.getInstance(conf, "Matrix Multiplication");
job.setJarByClass(MatrixMultiplication.class);
job.setMapperClass(MatrixMapper.class);
job.setReducerClass(MatrixReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(Text.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
```
注意,这只是一个简单的示例程序,实际应用中还需要考虑矩阵分块、矩阵压缩等技术,以减少计算量和网络传输量。
阅读全文
相关推荐
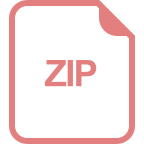
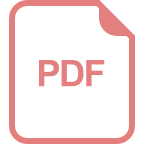














