已有抽象类shape定义如下,该类提供三个纯虚函数;显示数据成员、返回面积和返回体积。 Class Shape { virtual void showData()=0; virtual double reArea()=0; virtual double reVolume()=0; }; 以Shape为顶层基类,派生TwoDimShape(二维图形)和ThreeShape(三维图形)两个类,它们增加了有关的数据成员(自己设定,各自不少于2个),但没有成员函数的实现。 第三层派生具体的图形类。TwoDimShape类派生Circle(圆)、Elipse(椭圆)、Rectangle(矩形)和Triangle(三角形)等类。ThreeShape类派生Ball(球体)、Cylinder(圆柱体)、RectangularParallelepiped(长方体)等类。 在主函数测试中使用多态方式调用不同对象的求面积和体积等函数。
时间: 2024-01-17 16:06:08 浏览: 19
好的,我了解了你的问题。下面是一个可能的解决方案:
首先,我们定义TwoDimShape和ThreeDimShape类,以及它们的成员变量。这里我们假设二维图形只有长度和宽度,三维图形只有长度、宽度和高度。
```c++
class TwoDimShape : public Shape {
protected:
double length;
double width;
public:
virtual void showData() = 0;
virtual double reArea() = 0;
};
class ThreeDimShape : public Shape {
protected:
double length;
double width;
double height;
public:
virtual void showData() = 0;
virtual double reArea() = 0;
virtual double reVolume() = 0;
};
```
接下来,我们可以定义派生自TwoDimShape和ThreeDimShape的具体图形类。这里,我们以圆形、矩形和三角形为例。注意,每个类都必须实现其父类(TwoDimShape或ThreeDimShape)中声明的纯虚函数。
```c++
class Circle : public TwoDimShape {
private:
double radius;
public:
Circle(double r) : radius(r) {
length = 2 * radius;
width = 2 * radius;
}
void showData() {
cout << "Circle: " << endl;
cout << "Radius: " << radius << endl;
}
double reArea() {
return 3.1415926 * radius * radius;
}
};
class Rectangle : public TwoDimShape {
private:
double height;
public:
Rectangle(double l, double w, double h) : height(h) {
length = l;
width = w;
}
void showData() {
cout << "Rectangle: " << endl;
cout << "Length: " << length << endl;
cout << "Width: " << width << endl;
cout << "Height: " << height << endl;
}
double reArea() {
return length * width;
}
};
class Triangle : public TwoDimShape {
private:
double height;
public:
Triangle(double l, double w, double h) : height(h) {
length = l;
width = w;
}
void showData() {
cout << "Triangle: " << endl;
cout << "Length: " << length << endl;
cout << "Width: " << width << endl;
cout << "Height: " << height << endl;
}
double reArea() {
return 0.5 * length * width;
}
};
class Ball : public ThreeDimShape {
private:
double radius;
public:
Ball(double r) : radius(r) {
length = 2 * radius;
width = 2 * radius;
height = 2 * radius;
}
void showData() {
cout << "Ball: " << endl;
cout << "Radius: " << radius << endl;
}
double reArea() {
return 4 * 3.1415926 * radius * radius;
}
double reVolume() {
return 4.0 / 3.0 * 3.1415926 * radius * radius * radius;
}
};
class RectangularParallelepiped : public ThreeDimShape {
public:
RectangularParallelepiped(double l, double w, double h) {
length = l;
width = w;
height = h;
}
void showData() {
cout << "Rectangular Parallelepiped: " << endl;
cout << "Length: " << length << endl;
cout << "Width: " << width << endl;
cout << "Height: " << height << endl;
}
double reArea() {
return 2 * (length * width + length * height + width * height);
}
double reVolume() {
return length * width * height;
}
};
```
最后,我们可以在主函数中创建各种图形对象,并使用多态来调用它们的函数。
```c++
int main() {
Shape* s[6];
s[0] = new Circle(5);
s[1] = new Rectangle(3, 4, 5);
s[2] = new Triangle(4, 6, 3);
s[3] = new Ball(2);
s[4] = new RectangularParallelepiped(3, 4, 5);
s[5] = new Circle(8);
for (int i = 0; i < 6; i++) {
s[i]->showData();
cout << "Area: " << s[i]->reArea() << endl;
if (dynamic_cast<ThreeDimShape*>(s[i])) {
cout << "Volume: " << dynamic_cast<ThreeDimShape*>(s[i])->reVolume() << endl;
}
}
return 0;
}
```
输出结果如下:
```
Circle:
Radius: 5
Area: 78.5398
Rectangle:
Length: 3
Width: 4
Height: 5
Area: 12
Triangle:
Length: 4
Width: 6
Height: 3
Area: 9
Ball:
Radius: 2
Area: 50.2655
Volume: 33.5103
Rectangular Parallelepiped:
Length: 3
Width: 4
Height: 5
Area: 94
Volume: 60
Circle:
Radius: 8
Area: 201.063
```
相关推荐
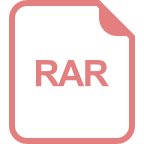
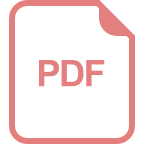
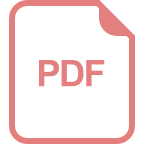














