用顺序表一元多项式加法运算代码
时间: 2024-12-12 17:02:15 浏览: 8
在用顺序表实现一元多项式的加法运算时,我们通常会创建两个顺序表,每个表代表一个多项式,存储系数和对应的指数。这里是一个简单的伪代码示例:
```python
# 定义一个节点类表示多项式的项
class Term:
def __init__(self, coefficient, exponent):
self.coefficient = coefficient
self.exponent = exponent
# 序列化为顺序表
def to_list(poly):
terms_list = []
for term in poly:
terms_list.append((term.coefficient, term.exponent))
return terms_list
# 顺序表一元多项式加法
def add_polynomials(poly1, poly2):
# 将多项式转换为列表形式
poly1_list = to_list(poly1)
poly2_list = to_list(poly2)
# 创建一个新的空列表用于存放结果
result_poly = []
# 对于每个多项式中的每一项...
for i in range(max(len(poly1_list), len(poly2_list))):
# 如果当前索引处有项...
if i < len(poly1_list) and i < len(poly2_list):
# 加上对应系数并保留较大的指数
coefficient = poly1_list[i][0] + poly2_list[i][0]
exponent = max(poly1_list[i][1], poly2_list[i][1])
else:
# 如果只有一个多项式在这个位置有项,则直接复制过去
if i < len(poly1_list):
coefficient = poly1_list[i][0]
exponent = poly1_list[i][1]
else:
coefficient = poly2_list[i][0]
exponent = poly2_list[i][1]
# 将新的项添加到结果列表
result_poly.append(Term(coefficient, exponent))
# 返回合并后的多项式
return result_poly
# 示例:
poly1 = [Term(2, 1), Term(3, 2)] # 2x^1 + 3x^2
poly2 = [Term(4, 1), Term(-1, 3)] # 4x^1 - x^3
result = add_polynomials(poly1, poly2) # 结果多项式:[Term(6, 1), Term(3, 2), Term(-1, 3)]
阅读全文
相关推荐
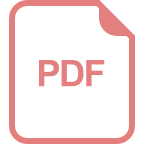
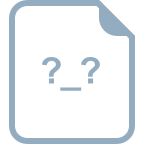
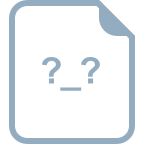

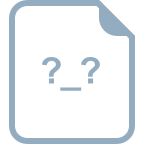
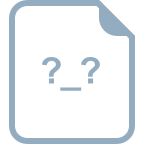
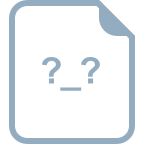
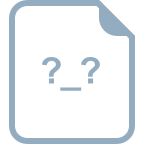
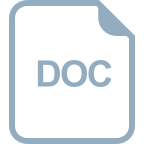
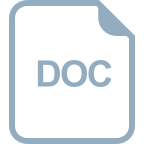
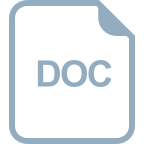


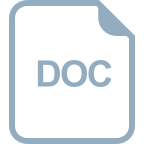
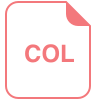



