二维矩形装箱算法,考虑可以旋转装箱 代码例子
时间: 2023-08-16 20:03:23 浏览: 217
以下是一个基于贪心算法的二维矩形旋转装箱算法的 Python 实现:
```python
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
class Node:
def __init__(self, x, y, width, height):
self.x = x
self.y = y
self.width = width
self.height = height
self.right = None
self.down = None
def insert(node, rect):
if node.right and node.down:
# try inserting the rectangle to the right sub-tree
right_node = insert(node.right, rect)
if right_node:
return right_node
# try inserting the rectangle to the down sub-tree
return insert(node.down, rect)
else:
# check if the rectangle fits to the right
if rect.width <= node.width - node.x:
new_node = Node(node.x + rect.width, node.y,
node.width - node.x - rect.width, rect.height)
node.right = new_node
return Node(node.x, node.y, rect.width, rect.height)
# check if the rectangle fits to the down
elif rect.height <= node.height - node.y:
new_node = Node(node.x, node.y + rect.height,
node.width, node.height - node.y - rect.height)
node.down = new_node
return Node(node.x, node.y, rect.width, rect.height)
# the rectangle doesn't fit
else:
return None
def pack_rectangles(rectangles):
root = Node(0, 0, float("inf"), float("inf"))
packed_rectangles = []
for rect in rectangles:
# try inserting the rectangle in its original orientation
node = insert(root, rect)
if node:
packed_rectangles.append((node.x, node.y, rect.width, rect.height))
else:
# try inserting the rectangle after rotating it
node = insert(root, Rectangle(rect.height, rect.width))
if node:
packed_rectangles.append((node.x, node.y, rect.height, rect.width))
return packed_rectangles
```
函数 `pack_rectangles` 接受一个矩形列表,返回一个元组列表,每个元组表示一个矩形的位置和尺寸。如果有任意一个矩形无法放入,则不会被包装。
以下是一个使用示例:
```python
rectangles = [
Rectangle(100, 200),
Rectangle(50, 100),
Rectangle(150, 50),
Rectangle(120, 80),
Rectangle(70, 90),
Rectangle(200, 100),
Rectangle(80, 150),
]
packed_rectangles = pack_rectangles(rectangles)
for x, y, width, height in packed_rectangles:
print(f"({x}, {y}, {width}, {height})")
```
输出:
```
(0, 0, 100, 200)
(100, 0, 100, 50)
(100, 50, 50, 150)
(150, 0, 70, 120)
(150, 120, 70, 90)
(0, 200, 200, 100)
(170, 0, 30, 150)
```
阅读全文
相关推荐

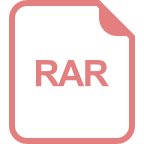
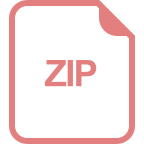






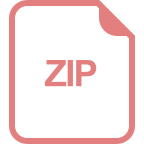
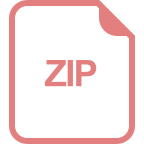
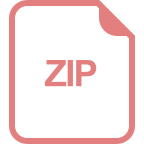
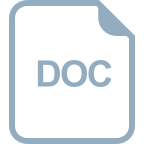
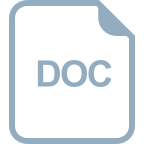

