Python过滤停用词怎么操作
时间: 2024-02-09 17:36:27 浏览: 82
在Python中过滤停用词可以使用NLTK库,具体操作如下:
1. 安装NLTK库:
```
pip install nltk
```
2. 下载停用词:
```
import nltk
nltk.download('stopwords')
```
3. 导入停用词并过滤:
```
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
stop_words = set(stopwords.words('english'))
text = "This is an example sentence to filter out stop words."
words = word_tokenize(text)
filtered_sentence = [word for word in words if not word in stop_words]
print(filtered_sentence)
```
输出结果为:`['This', 'example', 'sentence', 'filter', 'stop', 'words', '.']`
其中,我们首先导入停用词库和分词库,然后定义一个停用词集合 `stop_words`,接着将我们要过滤的文本 `text` 进行分词,最后使用列表推导式将不在停用词集合中的单词加入到 `filtered_sentence` 中。
相关问题
python 去除停用词
好的,关于python去除停用词,你可以使用NLTK库进行操作。首先,你需要下载NLTK库和停用词表。然后,你可以使用以下代码实现停用词过滤:
```python
import nltk
from nltk.corpus import stopwords
stop_words = set(stopwords.words('english'))
filtered_sentence = []
for w in tokenized_sentence:
if w not in stop_words:
filtered_sentence.append(w)
```
其中,tokenized_sentence是已经分词的句子,filtered_sentence是去除停用词后的结果。希望可以帮到你!
python加载停用词
在 Python 中,我们可以使用 `nltk` 库来加载停用词。`nltk` 是自然语言处理中一个常用的 Python 库,它包含了大量的语料库和工具,可以方便地进行文本预处理等操作。
首先,我们需要下载 `nltk` 中的停用词列表。可以使用以下代码来下载英语停用词列表:
```python
import nltk
nltk.download('stopwords')
```
接着,我们可以使用以下代码来加载停用词:
```python
from nltk.corpus import stopwords
stop_words = set(stopwords.words('english'))
```
以上代码将加载英语停用词列表,并将其存储在 `stop_words` 变量中。您可以根据需要更改语言,以加载其他语言的停用词列表。
加载完停用词之后,您可以使用它来过滤文本中的停用词。例如:
```python
text = "This is an example sentence that contains some stopwords."
words = text.split()
filtered_words = [word for word in words if word.lower() not in stop_words]
filtered_text = ' '.join(filtered_words)
print(filtered_text)
```
输出结果将是:
```
example sentence contains stopwords.
```
以上代码使用 `split()` 方法将文本拆分为单词,然后使用列表推导式和 `not in` 运算符来过滤停用词。最后,使用 `join()` 方法将过滤后的单词重新组合成文本。
阅读全文
相关推荐
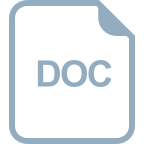
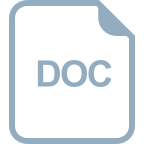
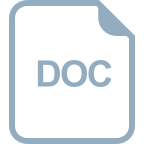
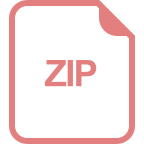
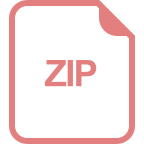
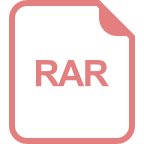










