使用stm32标准库 通过UART发送ascii码,发送内容为浮点数123.456
时间: 2024-05-03 09:22:39 浏览: 6
以下是使用STM32标准库通过UART发送浮点数的代码(使用printf函数):
```c
#include "stm32f10x.h"
#include <stdio.h>
void USART1_Init(void);
void USART1_SendChar(char ch);
void USART1_SendString(char* str);
int main(void)
{
float num = 123.456;
char buffer[20]; // 用于存储浮点数转换后的字符串
USART1_Init(); // 初始化USART1
// 将浮点数转换成字符串
sprintf(buffer, "%.3f", num);
// 发送字符串
USART1_SendString(buffer);
while (1)
{
// 主循环
}
}
void USART1_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
// 使能GPIOA和USART1的时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_USART1, ENABLE);
// 配置USART1的TX引脚(PA9)为复用推挽输出
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置USART1
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
// 使能USART1
USART_Cmd(USART1, ENABLE);
}
void USART1_SendChar(char ch)
{
// 等待发送缓冲区为空
while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
// 发送一个字符
USART_SendData(USART1, ch);
}
void USART1_SendString(char* str)
{
// 发送字符串
while (*str)
{
USART1_SendChar(*str++);
}
}
```
相关推荐
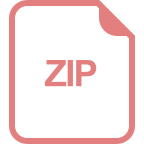














