yv_mask = torch.unsqueeze(yv[mask] + pred_offset_mask[..., 1], -1)
时间: 2024-04-09 07:31:16 浏览: 143
这是一个用于处理张量的操作。根据给定的掩码(mask),它选择了与掩码相对应位置为True的元素,并在维度上添加了一个额外的维度。然后,通过将pred_offset_mask的第二个维度添加到yv[mask]中,得到最终的结果yv_mask。请注意,这里使用了torch.unsqueeze函数来添加维度。
相关问题
def decode_outputs(self, outputs, dtype): grids = [] strides = [] for (hsize, wsize), stride in zip(self.hw, self.strides): yv, xv = torch.meshgrid([torch.arange(hsize), torch.arange(wsize)]) grid = torch.stack((xv, yv), 2).view(1, -1, 2) grids.append(grid) shape = grid.shape[:2] strides.append(torch.full((*shape, 1), stride)) grids = torch.cat(grids, dim=1).type(dtype) strides = torch.cat(strides, dim=1).type(dtype) outputs[..., :2] = (outputs[..., :2] + grids) * strides outputs[..., 2:4] = torch.exp(outputs[..., 2:4]) * strides return outputs 在GPU环境进行速度优化 并提供代码示例
以下是一个使用CUDA并行计算的示例代码:
```python
import torch
class Decoder:
def __init__(self, hw, strides):
self.hw = hw
self.strides = strides
def decode_outputs(self, outputs, dtype):
grids = []
strides = []
for (hsize, wsize), stride in zip(self.hw, self.strides):
yv, xv = torch.meshgrid([torch.arange(hsize), torch.arange(wsize)])
grid = torch.stack((xv, yv), 2).view(1, -1, 2)
grids.append(grid)
shape = grid.shape[:2]
strides.append(torch.full((*shape, 1), stride))
grids = torch.cat(grids, dim=1).type(dtype).cuda()
strides = torch.cat(strides, dim=1).type(dtype).cuda()
outputs = outputs.cuda()
outputs[..., :2] = (outputs[..., :2] + grids) * strides
outputs[..., 2:4] = torch.exp(outputs[..., 2:4]) * strides
return outputs.cpu()
```
这段代码与之前的代码基本相同,只是在计算 grids 和 strides 时,将其转换为 CUDA 张量,并使用 GPU 进行计算。同时将模型输出 outputs 也转换为 CUDA 张量,并在计算后将其转换回 CPU 张量。这样可以在 GPU 环境下采用并行思维进行速度优化,提高代码的执行效率。
def decode_outputs(self, outputs, dtype): grids = [] strides = [] for (hsize, wsize), stride in zip(self.hw, self.strides): yv, xv = torch.meshgrid([torch.arange(hsize, dtype=dtype), torch.arange(wsize, dtype=dtype)]) grid = torch.stack((xv, yv), dim=2).view(1, -1, 2) grids.append(grid) shape = grid.shape[:2] strides.append(torch.full((*shape, 1), stride, dtype=dtype)) grids = torch.cat(grids, dim=1) strides = torch.cat(strides, dim=1) outputs[..., :2].add_(grids).mul_(strides) outputs[..., 2:4].exp_().mul_(strides) return outputs通过张量列表的形式替换for循环速度优化并提供代码
def decode_outputs(self, outputs, dtype):
hw = self.hw
strides = self.strides
grids = [torch.stack((torch.meshgrid([torch.arange(hsize, dtype=dtype), torch.arange(wsize, dtype=dtype)])), dim=2).view(1, -1, 2) for (hsize, wsize) in hw]
grids = torch.cat(grids, dim=1)
strides = torch.cat([torch.full((*grid.shape[:2], 1), stride, dtype=dtype) for stride, grid in zip(strides, grids)], dim=1)
outputs[..., :2] = (outputs[..., :2] + grids) * strides
outputs[..., 2:4] = torch.exp(outputs[..., 2:4]) * strides
return outputs
阅读全文
相关推荐
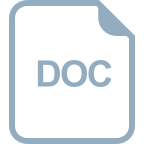
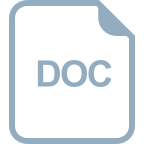
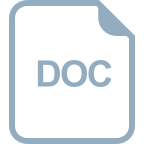





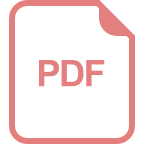
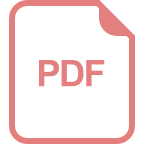




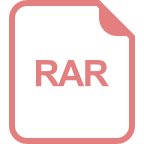
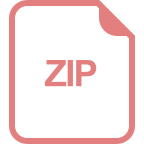
