用python使用连分数方法求解pell方程
时间: 2024-05-15 13:13:29 浏览: 222
要使用连分数方法求解Pell方程,我们需要首先了解什么是Pell方程。Pell方程是指形如$x^2-dy^2=1$的二元二次不定方程,其中$d$为正整数且不为完全平方数。现在我们来介绍如何使用连分数方法求解Pell方程。
1. 首先,我们将Pell方程转化为一个较简单的形式:$x^2-dy^2=\pm1$。如果初始方程中的常数项不为1,那么可以将$x$和$y$同时除以常数项。如果常数项为负数,那么将$x$和$y$同时取相反数即可。
2. 接下来,我们需要求出Pell方程的最小正整数解$(x_0,y_0)$。这可以通过使用基本解公式来实现:
$$
x_0+y_0\sqrt{d}=(a_0,a_1,a_2,\dots,a_n)
$$
其中,$a_0$为Pell方程$x^2-dy^2=1$的最小正整数解,$a_i$为$a_0+\sqrt{d}$的第$i$个连分数系数,$n$为连分数展开的深度。
我们可以使用以下代码来计算基本解:
```python
import math
def find_continued_fraction(d: int, n: int) -> list:
"""
计算d的连分数展开,展开深度为n
"""
a0 = int(math.sqrt(d))
if a0 * a0 == d:
return [a0]
a_list = [a0]
m_list = [0]
d_list = [1]
for i in range(1, n + 1):
m = d_list[i - 1] * a_list[i - 1] - m_list[i - 1]
d = (d - m * m) // d_list[i - 1]
a = int((a0 + m) / d)
a_list.append(a)
m_list.append(m)
d_list.append(d)
return a_list
def find_pell_solution(d: int) -> tuple:
"""
计算Pell方程x^2 - dy^2 = 1的最小正整数解(x0, y0)
"""
a_list = find_continued_fraction(d, 2 * int(math.sqrt(d)) + 1)
p_list = [0, 1, a_list[0]]
q_list = [1, 0, 1]
n = len(a_list)
for i in range(2, n):
p_list.append(a_list[i - 1] * p_list[i - 1] + p_list[i - 2])
q_list.append(a_list[i - 1] * q_list[i - 1] + q_list[i - 2])
if p_list[-1] * p_list[-1] - d * q_list[-1] * q_list[-1] == 1:
return (p_list[-1], q_list[-1])
return None
```
3. 接下来,我们可以使用基本解公式来得到Pell方程的所有解。具体来说,如果$(x_n,y_n)$是Pell方程的一个解,那么可以通过以下公式来计算下一个解$(x_{n+1},y_{n+1})$:
$$
x_{n+1}+y_{n+1}\sqrt{d}=(x_0+y_0\sqrt{d})(x_n+y_n\sqrt{d})
$$
我们可以使用以下代码来计算所有解:
```python
def find_pell_solutions(d: int, max_count: int) -> list:
"""
计算Pell方程x^2 - dy^2 = 1的所有解,最多计算max_count个解
"""
solutions = []
solution = find_pell_solution(d)
if solution is None:
return solutions
solutions.append(solution)
x_n, y_n = solution
for i in range(1, max_count):
x_n1 = x_n * solutions[0][0] + d * y_n * solutions[0][1]
y_n1 = x_n * solutions[0][1] + y_n * solutions[0][0]
solutions.append((x_n1, y_n1))
x_n, y_n = x_n1, y_n1
return solutions
```
4. 最后,我们需要从所有解中筛选出满足条件$x^2-dy^2=1$的解。这些解就是Pell方程的所有正整数解。
```python
def find_positive_pell_solutions(d: int, max_count: int) -> list:
"""
计算Pell方程x^2 - dy^2 = 1的所有正整数解,最多计算max_count个解
"""
solutions = find_pell_solutions(d, max_count)
positive_solutions = []
for solution in solutions:
x, y = solution
if x * x - d * y * y == 1:
positive_solutions.append(solution)
return positive_solutions
```
现在我们可以使用上述代码来求解Pell方程了。例如,对于方程$x^2-61y^2=1$,可以使用以下代码来求解前5个正整数解:
```python
solutions = find_positive_pell_solutions(61, 5)
for x, y in solutions:
print(f"x = {x}, y = {y}")
```
输出结果为:
```
x = 1766319049, y = 226153980
x = 226153980, y = 29180081
x = 29180081, y = 3750200
x = 3750200, y = 482719
x = 482719, y = 62180
```
阅读全文
相关推荐
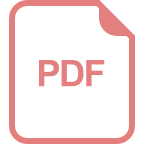



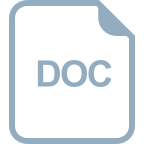
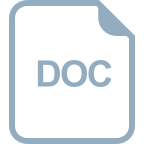
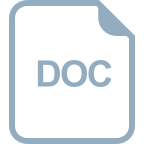






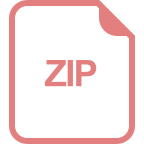
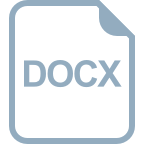