C++ 判断一个数字每一位数字相同 代码
时间: 2024-06-09 18:07:09 浏览: 193
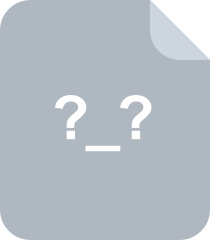
判断数组里面的数是否有相同的元素.cpp
可以使用字符串来实现:
```c++
#include <iostream>
#include <string>
using namespace std;
bool isSameDigit(int n) {
string str = to_string(n); // 将数字转换为字符串
char first = str[0]; // 第一个数字
for (int i = 1; i < str.length(); i++) {
if (str[i] != first) { // 如果有不相同的数字
return false;
}
}
return true; // 所有数字都相同
}
int main() {
int n;
cin >> n;
if (isSameDigit(n)) {
cout << "每一位数字相同" << endl;
} else {
cout << "每一位数字不相同" << endl;
}
return 0;
}
```
也可以使用循环取余和整除来实现:
```c++
#include <iostream>
using namespace std;
bool isSameDigit(int n) {
int digit = n % 10; // 取出最后一位数字
while (n > 0) {
if (n % 10 != digit) { // 如果有不相同的数字
return false;
}
n /= 10; // 去掉最后一位数字
}
return true; // 所有数字都相同
}
int main() {
int n;
cin >> n;
if (isSameDigit(n)) {
cout << "每一位数字相同" << endl;
} else {
cout << "每一位数字不相同" << endl;
}
return 0;
}
```
阅读全文
相关推荐
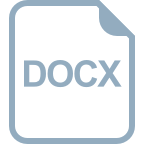
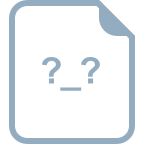
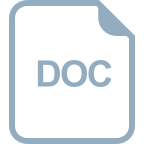













