用C语言编写一个函数,把一个unsigned int类型值中的所有位向左旋转指定的位。例如,rotate_1(x,4)把x中所有位向左移动4个位置,而且从最左端移出的位会重新出现在右端。也就是说,把从高阶位移出的位放入低阶位。在一个程序中测试该函数。
时间: 2024-02-21 20:02:14 浏览: 26
好的,这是你的第二个问题,以下是函数的代码实现:
```c
#include <stdio.h>
unsigned int rotate_left(unsigned int x, int n) {
int bits = sizeof(unsigned int) * 8;
n %= bits;
return (x << n) | (x >> (bits - n));
}
int main() {
unsigned int x = 0x12345678;
int n = 4;
printf("0x%x rotated left by %d bits is 0x%x\n", x, n, rotate_left(x, n));
return 0;
}
```
这个函数接受两个参数:一个unsigned int类型的值x和一个整数n。它将x中的所有位向左旋转n个位置,并返回结果。从最左端移出的位会重新出现在右端,也就是说,把从高阶位移出的位放入低阶位。
例如,如果你想将0x12345678中的所有位向左移动4个位置,你可以调用函数rotate_left(x, 4)。函数将返回0x23456781。
你可以在main函数中测试该函数,例如:
```c
unsigned int x = 0x12345678;
int n = 4;
printf("0x%x rotated left by %d bits is 0x%x\n", x, n, rotate_left(x, n));
```
输出结果应该是:
```
0x12345678 rotated left by 4 bits is 0x23456781
```
希望这个函数能够帮助你。如果你有任何问题或疑问,请随时提出。
相关推荐
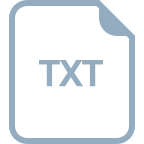














