conversion from double to int
时间: 2023-04-23 11:05:23 浏览: 355
将double转换为int的方法是使用强制类型转换符号,即将double值放在括号内,前面加上int,例如:
double d = 3.14;
int i = (int)d;
这将把d的值转换为整数,并将其存储在i中。请注意,这将截断小数部分,因此在进行此转换时应谨慎。
相关问题
Clang-Tidy: Narrowing conversion from 'double' to 'int'
This warning message from Clang-Tidy indicates that there is a potential narrowing conversion from a double type to an int type. A narrowing conversion means that some information might be lost during the conversion.
In C++, a double is a floating-point type that can represent decimal numbers with a higher precision compared to an int, which is an integer type. When you assign a double value to an int variable, the compiler may issue a warning because the fractional part of the double value will be truncated, losing precision.
To resolve this warning, you have a few options:
1. If you are intentionally truncating the fractional part, you can use a static_cast to explicitly convert the double value to an int:
```cpp
double myDouble = 3.14;
int myInt = static_cast<int>(myDouble);
```
2. If you want to perform a rounding operation, you can use the round or nearbyint functions from the `<cmath>` library:
```cpp
#include <cmath>
double myDouble = 3.14;
int myInt = std::round(myDouble); // or std::nearbyint(myDouble)
```
3. If you want to avoid the narrowing conversion altogether, you can choose a different type that can represent decimal numbers with higher precision, such as float or double.
It's important to note that the appropriate solution depends on your specific use case and the desired behavior of your program.
monitorsystem.cpp:292:5: error: no matching function for call to 'number' qstring.h:757:20: note: candidate function not viable: no known conversion from 'quint8 [6]' to 'int' for 1st argument qstring.h:758:20: note: candidate function not viable: no known conversion from 'quint8 [6]' to 'uint' (aka 'unsigned int') for 1st argument qstring.h:759:20: note: candidate function not viable: no known conversion from 'quint8 [6]' to 'long' for 1st argument qstring.h:760:20: note: candidate function not viable: no known conversion from 'quint8 [6]' to 'ulong' (aka 'unsigned long') for 1st argument qstring.h:761:20: note: candidate function not viable: no known conversion from 'quint8 [6]' to 'qlonglong' (aka 'long long') for 1st argument qstring.h:762:20: note: candidate function not viable: no known conversion from 'quint8 [6]' to 'qulonglong' (aka 'unsigned long long') for 1st argument qstring.h:763:20: note: candidate function not viable: no known conversion from 'quint8 [6]' to 'double' for 1st argument
这个错误提示是因为在调用 QString::number() 函数时,将一个 quint8 数组作为参数传递给了该函数,但是 QString::number() 函数没有接受 quint8 数组作为参数的重载函数。
如果要将 quint8 数组转换为 QString 类型,可以使用 QByteArray 类型的 fromRawData() 函数和 QString 的构造函数。具体做法如下:
```
quint8 a[6] = {1, 2, 3, 4, 5, 6};
int size = sizeof(quint8) * 6;
QString result = QString::fromUtf8(QByteArray::fromRawData(reinterpret_cast<const char*>(a), size), size);
// result 的值为 ""
```
在这个例子中,fromRawData() 函数接受两个参数:一个指向字符数组的指针和数组的大小。首先将 quint8 数组转换为 char 数组的指针,然后使用该指针和数组的大小作为参数调用 fromRawData() 函数,得到一个 QByteArray 类型的对象。接着使用 QString 的构造函数将 QByteArray 类型的对象转换为 QString 类型的字符串。需要注意的是,构造函数的第二个参数指定的是字符串的长度。在这个例子中,由于 quint8 数组的大小为 6,因此字符串的长度也应该为 6。
相关推荐
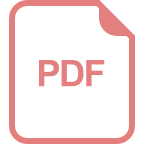
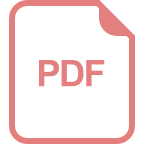
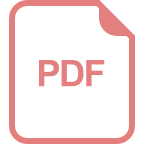












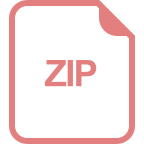