ROS里的休眠函数
时间: 2023-10-03 20:05:23 浏览: 61
在ROS中,可以使用`ros::Duration`类来实现休眠功能。该类可用于表示一段时间,单位为秒和纳秒。要使程序休眠一段时间,可以使用`ros::Duration`类的`sleep()`函数。
以下是一个示例代码片段,演示如何在ROS中使用`ros::Duration`类的`sleep()`函数来休眠程序:
```
#include <ros/ros.h>
#include <ros/duration.h>
int main(int argc, char **argv)
{
ros::init(argc, argv, "example_node");
ros::NodeHandle nh;
// Sleep for 5 seconds
ros::Duration(5.0).sleep();
return 0;
}
```
在上面的代码中,我们使用`ros::Duration`类的构造函数来创建一个表示5秒的`ros::Duration`对象,然后调用它的`sleep()`函数来休眠程序。注意,这里我们没有使用`ros::Rate`类,因为我们只需要在程序中停留一段固定的时间,而不需要控制程序的运行速度。
相关问题
ROS publish函数
ROS中的publish函数用于将消息发布到指定的主题上。它通常由ROS节点中的publisher对象调用。下面是一个简单的python代码示例:
```python
import rospy
from std_msgs.msg import String
rospy.init_node('my_publisher') # 初始化节点
pub = rospy.Publisher('my_topic', String, queue_size=10) # 创建publisher对象
rate = rospy.Rate(10) # 设置发布频率为10Hz
while not rospy.is_shutdown():
msg = String() # 创建消息对象
msg.data = 'Hello, world!' # 设置消息内容
pub.publish(msg) # 发布消息
rate.sleep() # 按照设置的频率休眠
```
上述代码中,首先通过`rospy.init_node()`函数初始化了一个名为`my_publisher`的节点。然后,通过`rospy.Publisher()`函数创建了一个名为`my_topic`的主题,消息类型为`std_msgs/String`,队列大小为10,并将其绑定到一个名为`pub`的publisher对象上。接下来,通过`rospy.Rate()`函数设置了发布频率为10Hz,然后在一个循环中不断地发布消息,直到节点被关闭。在每次循环中,首先创建了一个空的`std_msgs/String`消息对象,然后设置了其数据成员`data`为`'Hello, world!'`,最后通过`pub.publish()`函数将消息发布出去。发布完消息后,通过`rate.sleep()`函数让程序休眠一段时间,以保证发布频率达到设定的值。
在ros中 waitForTransform
在ROS中,`waitForTransform()`函数是TF库中的一个函数,用于等待两个帧之间的转换可用。它通常用于等待两个帧之间的转换被广播,以便您可以使用`lookupTransform()`函数来查询它们之间的转换。
这个函数的语法如下:
```cpp
bool tf_listener.waitForTransform(const std::string& target_frame, const std::string& source_frame, const ros::Time& time, const ros::Duration& timeout, const ros::Duration& polling_sleep_duration);
```
其中,`target_frame`和`source_frame`是要查询的两个帧的名称,`time`是您想要查询的时间戳,`timeout`是等待时间的最大值,`polling_sleep_duration`是每次查询之间的休眠时间。
如果转换在超时之前变得可用,则函数将返回`true`,否则将返回`false`。
以下是一个使用`waitForTransform()`函数的示例代码,用于等待`base_link`和`laser_link`帧之间的转换:
```cpp
#include <tf/transform_listener.h>
#include <ros/ros.h>
int main(int argc, char** argv)
{
ros::init(argc, argv, "tf_listener");
ros::NodeHandle nh;
tf::TransformListener tf_listener;
std::string target_frame = "base_link";
std::string source_frame = "laser_link";
ros::Time time = ros::Time(0);
ros::Duration timeout = ros::Duration(5.0);
bool success = tf_listener.waitForTransform(target_frame, source_frame, time, timeout, ros::Duration(0.01));
if (success) {
ROS_INFO("Transform from %s to %s is available", source_frame.c_str(), target_frame.c_str());
} else {
ROS_ERROR("Failed to get transform from %s to %s", source_frame.c_str(), target_frame.c_str());
}
return 0;
}
```
在这个例子中,我们将等待5秒钟来获取`base_link`和`laser_link`帧之间的转换,并在转换可用时输出一条消息。
相关推荐
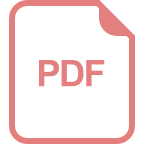
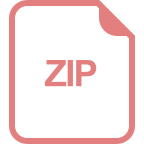












