读取 const unsigned char*数据
时间: 2023-09-18 15:11:43 浏览: 144
可以使用指针来读取 const unsigned char* 类型的数据。这种数据类型通常代表一个指向无符号字符数组的指针,也就是一个指向内存中连续的无符号字符数据的指针。
下面是一个简单的示例代码,演示如何读取 const unsigned char* 类型的数据:
```c++
const unsigned char* data = ...; // 假设已经有数据了
int data_len = ...; // 假设已经知道数据长度了
// 逐个读取每个字符数据
for (int i = 0; i < data_len; i++) {
unsigned char ch = *(data + i); // 读取第 i 个字符数据
// 处理字符数据 ch
}
```
在上面的代码中,我们使用指针加上索引的方式来访问 const unsigned char* 类型的数据。具体来说,`*(data + i)` 表示指针 data 加上偏移量 i 后所指向的地址处的数据,即第 i 个字符数据。
需要注意的是,由于 const unsigned char* 类型的数据通常表示一个字符串,因此可以使用字符串函数来处理该数据。例如,可以使用 `strlen` 函数获取字符串长度,使用 `strcmp` 函数比较两个字符串是否相等等。
相关问题
const unsigned char*
const unsigned char* 是一个指向无符号字符常量的指针。这意味着它指向的内存中存储的数据是无符号字符类型的,并且在声明后不能修改。使用该指针可以读取该内存中的无符号字符数据。根据引用中的解释,无符号字符数据传递给printf函数时会被提升为unsigned int类型,并且不进行符号位扩展。根据引用中的解释,如果使用%x格式化输出无符号字符数据时,记得要将其转换为无符号类型,以避免产生意外的变化。<span class="em">1</span><span class="em">2</span>
请使用JPEG_LS算法针对8*8大小的ARGB像素数据块进行无损压缩和解压. 完善以下解压、压缩函数,其中pClrBlk为原始数据块,pTile为压缩后的数据块 int argb2tile(const unsigned char* pClrBlk, unsigned char* pTile, int* pTileSize) int tile2argb(const unsigned char* pTile, int nTileSize, unsigned char* pClrBlk)
由于JPEG_LS算法是基于预测误差的无损压缩算法,我们需要先对ARGB像素数据块进行预测,然后计算预测误差,最后对误差进行编码。
以下是完善后的压缩和解压函数:
```c++
const int MAX_VAL = 255; // 最大像素值
const int NEAR = 0; // 预测模式为NEAR
const int LINEAR = 1; // 预测模式为LINEAR
// 将8位无符号整数转为有符号整数
int signedByte(unsigned char x) {
if (x > MAX_VAL / 2) return x - MAX_VAL - 1;
else return x;
}
// 将有符号整数转为8位无符号整数
unsigned char unsignedByte(int x) {
if (x < 0) return MAX_VAL + x + 1;
else return x;
}
// 计算预测误差
int predict(int mode, int a, int b, int c) {
if (mode == NEAR) return a - b;
else if (mode == LINEAR) return a + b - c;
else return 0;
}
// 将一个8*8大小的ARGB像素数据块压缩为一个数据块
int argb2tile(const unsigned char* pClrBlk, unsigned char* pTile, int* pTileSize) {
int mode = NEAR; // 默认预测模式为NEAR
int x, y, c;
int pred, diff;
int nearA, nearB, linearA, linearB, linearC;
// 压缩后的数据块的第一个字节存储预测模式
pTile[0] = mode;
int k = 1; // 压缩后数据的索引
// 压缩每个像素
for (x = 0; x < 8; x++) {
for (y = 0; y < 8; y++) {
c = y * 4 + x * 32; // 每个像素的索引
if (x == 0 && y == 0) { // 第一个像素直接记录ARGB值
for (int i = 0; i < 4; i++) {
pTile[k++] = pClrBlk[c + i];
}
}
else { // 其他像素先预测,再计算误差
nearA = signedByte(pClrBlk[c - 4]); // 左边像素的A值
nearB = signedByte(pClrBlk[c - 4 + 1]); // 左边像素的R值
pred = predict(mode, nearA, nearB, 0); // 预测当前像素的R值
diff = signedByte(pClrBlk[c + 1]) - pred; // 计算R值的误差
pTile[k++] = unsignedByte(pred); // 存储预测结果
pTile[k++] = unsignedByte(diff); // 存储误差值
linearA = signedByte(pClrBlk[c - 4 * 3]); // 上面像素的A值
linearB = signedByte(pClrBlk[c - 4 * 3 + 1]); // 上面像素的R值
linearC = signedByte(pClrBlk[c - 4]); // 左边像素的R值
pred = predict(mode, linearA, linearB, linearC); // 预测当前像素的G值
diff = signedByte(pClrBlk[c + 2]) - pred; // 计算G值的误差
pTile[k++] = unsignedByte(pred); // 存储预测结果
pTile[k++] = unsignedByte(diff); // 存储误差值
pred = predict(mode, linearA, linearB, 0); // 预测当前像素的B值
diff = signedByte(pClrBlk[c + 3]) - pred; // 计算B值的误差
pTile[k++] = unsignedByte(pred); // 存储预测结果
pTile[k++] = unsignedByte(diff); // 存储误差值
}
}
}
*pTileSize = k; // 存储压缩后数据的大小
return 0;
}
// 将一个压缩后的数据块解压为一个8*8大小的ARGB像素数据块
int tile2argb(const unsigned char* pTile, int nTileSize, unsigned char* pClrBlk) {
int mode = pTile[0]; // 获取预测模式
int x, y, c;
int pred, diff;
int nearA, nearB, linearA, linearB, linearC;
// 解压每个像素
for (x = 0; x < 8; x++) {
for (y = 0; y < 8; y++) {
c = y * 4 + x * 32; // 每个像素的索引
if (x == 0 && y == 0) { // 第一个像素直接记录ARGB值
for (int i = 0; i < 4; i++) {
pClrBlk[c + i] = pTile[1 + i];
}
}
else { // 其他像素先预测,再计算误差
nearA = signedByte(pClrBlk[c - 4]); // 左边像素的A值
nearB = signedByte(pClrBlk[c - 4 + 1]); // 左边像素的R值
pred = predict(mode, nearA, nearB, 0); // 预测当前像素的R值
diff = signedByte(pTile[1 + (x * 8 + y - 1) * 4 + 1]); // 读取R值的误差
pClrBlk[c + 1] = unsignedByte(pred + diff); // 计算并记录R值
linearA = signedByte(pClrBlk[c - 4 * 3]); // 上面像素的A值
linearB = signedByte(pClrBlk[c - 4 * 3 + 1]); // 上面像素的R值
linearC = signedByte(pClrBlk[c - 4]); // 左边像素的R值
pred = predict(mode, linearA, linearB, linearC); // 预测当前像素的G值
diff = signedByte(pTile[1 + (x * 8 + y - 1) * 4 + 2]); // 读取G值的误差
pClrBlk[c + 2] = unsignedByte(pred + diff); // 计算并记录G值
pred = predict(mode, linearA, linearB, 0); // 预测当前像素的B值
diff = signedByte(pTile[1 + (x * 8 + y - 1) * 4 + 3]); // 读取B值的误差
pClrBlk[c + 3] = unsignedByte(pred + diff); // 计算并记录B值
}
}
}
return 0;
}
```
注意,在压缩过程中,我们将每个像素的ARGB值分别存储,因此压缩后的数据块大小为8x8x4=256字节。在解压过程中,我们需要根据压缩后的数据索引计算出对应像素的ARGB值。
相关推荐
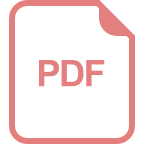
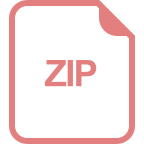
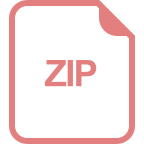













