Define a Python function calculate_investment(P,r,n,t) that calculates and returns the final amount after t years (Rounded to 2 decimal points). Where the principal amount P, rate of interest r , number of times the interest is compounded and number of years t are function parameters in order. Example 1 of function use print(calculate_investment(10000, 5,1,10)) Example 1 output 16288.95 Example 2 of function use print(calculate_investment(500, 10,4,5)) Example 2 output 819.31 Example 3 of function use print(calculate_investment(5000, 3,2,20)) Example 3 output 9070.09
时间: 2023-12-02 13:03:05 浏览: 28
Here's the implementation of the requested function:
```python
def calculate_investment(P, r, n, t):
# Calculate the interest rate per compounding period
rate = r / (100 * n)
# Calculate the total number of compounding periods
periods = n * t
# Calculate the final amount
amount = P * (1 + rate) ** periods
# Round the result to 2 decimal points and return it
return round(amount, 2)
```
Explanation:
- We first calculate the interest rate per compounding period by dividing the annual interest rate `r` by `100` and multiplying it by the number of times the interest is compounded `n`.
- We then calculate the total number of compounding periods by multiplying the number of times the interest is compounded `n` by the number of years `t`.
- Finally, we calculate the final amount by multiplying the principal amount `P` by `(1 + rate)` raised to the power of the total number of compounding periods `periods`.
We then round the result to 2 decimal points using the `round` function and return it.
相关推荐
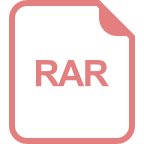
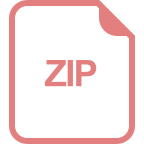














