基于深度优先搜索,设计C语言算法,判断一个无向图是否为一棵树 ,并给出完整代码
时间: 2023-08-22 19:05:17 浏览: 114
下面是基于深度优先搜索的 C 语言算法,用于判断一个无向图是否为一棵树。
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define MAX_VERTICES 100
// Graph structure using adjacency list
struct Graph {
int V;
struct Node* adjList[MAX_VERTICES];
};
// Node structure for adjacency list
struct Node {
int dest;
struct Node* next;
};
// Create a new node
struct Node* createNode(int dest) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->dest = dest;
newNode->next = NULL;
return newNode;
}
// Create a graph with V vertices
struct Graph* createGraph(int V) {
struct Graph* graph = (struct Graph*)malloc(sizeof(struct Graph));
graph->V = V;
for (int i = 0; i < V; i++) {
graph->adjList[i] = NULL;
}
return graph;
}
// Add an edge between two vertices
void addEdge(struct Graph* graph, int src, int dest) {
struct Node* newNode = createNode(dest);
newNode->next = graph->adjList[src];
graph->adjList[src] = newNode;
newNode = createNode(src);
newNode->next = graph->adjList[dest];
graph->adjList[dest] = newNode;
}
// Check if there is a cycle in the graph
bool isCyclic(struct Graph* graph, int v, bool visited[], int parent) {
visited[v] = true;
for (struct Node* i = graph->adjList[v]; i != NULL; i = i->next) {
int dest = i->dest;
if (!visited[dest]) {
if (isCyclic(graph, dest, visited, v)) {
return true;
}
} else if (dest != parent) {
return true;
}
}
return false;
}
// Check if the graph is a tree
bool isTree(struct Graph* graph) {
bool visited[MAX_VERTICES] = { false };
// Check if there is a cycle in the graph
if (isCyclic(graph, 0, visited, -1)) {
return false;
}
// Check if all vertices are visited (i.e. connected graph)
for (int i = 0; i < graph->V; i++) {
if (!visited[i]) {
return false;
}
}
return true;
}
// Example usage
int main() {
struct Graph* graph = createGraph(5);
addEdge(graph, 1, 0);
addEdge(graph, 0, 2);
addEdge(graph, 2, 1);
addEdge(graph, 0, 3);
addEdge(graph, 3, 4);
if (isTree(graph)) {
printf("Graph is a tree\n");
} else {
printf("Graph is not a tree\n");
}
return 0;
}
```
这里使用了邻接表来存储图,`isCyclic()` 方法用来检查从节点 `v` 开始是否存在环,`isTree()` 方法则用来检查整个图是否是一棵树。
阅读全文
相关推荐

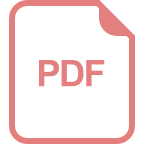
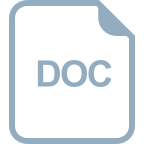
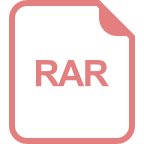
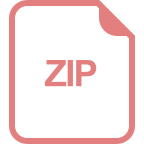
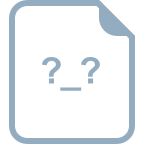
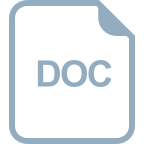
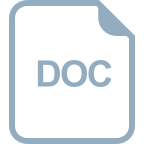
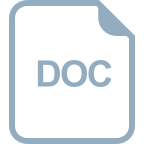
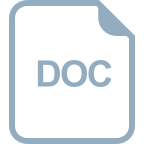
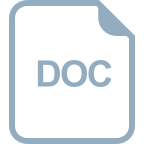
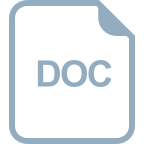
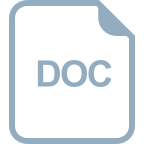
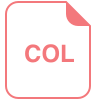
