new_x_shape = x.size()[:-1] + (self.num_attention_heads, self.attention_head_size)
时间: 2024-03-31 22:36:53 浏览: 56
这条代码的作用是将输入张量 `x` 的最后一个维度重新组合成一个新的维度元组,其中新的最后两个维度是 `self.num_attention_heads` 和 `self.attention_head_size`,以便进行多头注意力计算。具体来说, `x.size()[:-1]` 表示去掉 `x` 张量的最后一个维度后得到的维度元组,然后将其与 `(self.num_attention_heads, self.attention_head_size)` 拼接在一起得到新的维度元组 `new_x_shape`。
相关问题
import torchimport torch.nn as nnclass MultiHeadAttention(nn.Module): def __init__(self, d_model, num_heads): super(MultiHeadAttention, self).__init__() self.num_heads = num_heads self.d_model = d_model assert d_model % self.num_heads == 0 self.depth = d_model // self.num_heads self.Wq = nn.Linear(d_model, d_model) self.Wk = nn.Linear(d_model, d_model) self.Wv = nn.Linear(d_model, d_model) self.fc = nn.Linear(d_model, d_model) def scaled_dot_product_attention(self, Q, K, V, mask=None): d_k = Q.size(-1) scores = torch.matmul(Q, K.transpose(-1, -2)) / torch.sqrt(torch.tensor(d_k, dtype=torch.float32)) if mask is not None: scores = scores.masked_fill(mask == 0, -1e9) attention = torch.softmax(scores, dim=-1) output = torch.matmul(attention, V) return output, attention def split_heads(self, x, batch_size): x = x.view(batch_size, -1, self.num_heads, self.depth) return x.permute(0, 2, 1, 3) def forward(self, Q, K, V, mask=None): batch_size = Q.size(0) Q = self.Wq(Q) K = self.Wk(K) V = self.Wv(V) Q = self.split_heads(Q, batch_size) K = self.split_heads(K, batch_size) V = self.split_heads(V, batch_size) scaled_attention, attention = self.scaled_dot_product_attention(Q, K, V, mask) scaled_attention = scaled_attention.permute(0, 2, 1, 3).contiguous() scaled_attention = scaled_attention.view(batch_size, -1, self.d_model) output = self.fc(scaled_attention) return output, attention
上述代码是一个用PyTorch实现的多头注意力机制(Multi-Head Attention)的模块,该模块可以被用来构建神经网络模型。它的参数有:
- d_model:表示输入向量的维度,也就是embedding的维度。
- num_heads:表示attention头的数量。
输入的维度是:
- Q, K, V:三个输入张量的维度都为 [batch_size, seq_length, d_model],其中batch_size代表batch的大小,seq_length代表输入序列的长度,d_model代表输入向量的维度。
- mask:一个shape为[batch_size, 1, seq_length, seq_length]的张量,用于掩盖无效的位置,使得在计算注意力矩阵时,注意力矩阵中无效位置对应的权重为0。如果没有无效位置,则可以设置为None。
x, _ = F.multi_head_attention_forward( query=x, key=x, value=x, embed_dim_to_check=x.shape[-1], num_heads=self.num_heads, q_proj_weight=self.q_proj.weight, k_proj_weight=self.k_proj.weight, v_proj_weight=self.v_proj.weight, in_proj_weight=None, in_proj_bias=torch.cat([self.q_proj.bias, self.k_proj.bias, self.v_proj.bias]), bias_k=None, bias_v=None, add_zero_attn=False, dropout_p=0, out_proj_weight=self.c_proj.weight, out_proj_bias=self.c_proj.bias, use_separate_proj_weight=True, training=self.training, need_weights=False )
这段代码是一个多头注意力机制的前向传播实现,输入的是查询(query)、键(key)和值(value)的张量x,以及一些权重参数和超参数。在多头注意力机制中,将x分别进行线性变换并分成多个头,然后对每个头进行单独的注意力计算,并将计算结果在最后进行线性变换得到最终输出。这段代码中的参数设置和调用方式与PyTorch的API有些相似,但具体实现可能与PyTorch的实现略有不同。
阅读全文
相关推荐
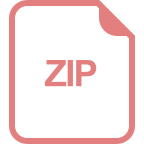
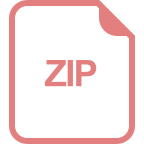
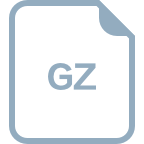













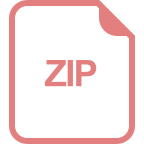
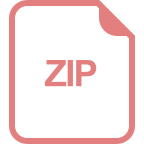