data_X = np.transpose(images, (0, 3, 2, 1)) data_Y = np.transpose(images, (0, 3, 2, 1))
时间: 2023-10-11 11:12:16 浏览: 30
这段代码中,np.transpose函数是用来对数组进行转置操作的。其中,第一个参数是需要进行转置的数组,第二个参数是一个元组,用来指定转置后的维度顺序。在这个例子中,对于数组images,第一维是样本数,第二维是通道数,第三维是高度,第四维是宽度。通过将元组设置为(0, 3, 2, 1),表示将原数组的第一维保持不变,将第二维和第四维交换位置,将第三维和第四维交换位置,最终得到的转置后的数组data_X和data_Y的维度顺序是样本数、宽度、高度、通道数,这种顺序在某些机器学习模型中比较常见。
相关问题
import pickle import numpy as np import os # from scipy.misc import imread def load_CIFAR_batch(filename): with open(filename, 'rb') as f: datadict = pickle.load(f, encoding='bytes') X = datadict[b'data'] Y = datadict[b'labels'] X = X.reshape(10000, 3, 32, 32).transpose(0, 2, 3, 1).astype("float") Y = np.array(Y) return X, Y def load_CIFAR10(ROOT): xs = [] ys = [] for b in range(1, 2): f = os.path.join(ROOT, 'data_batch_%d' % (b,)) X, Y = load_CIFAR_batch(f) xs.append(X) ys.append(Y) Xtr = np.concatenate(xs) Ytr = np.concatenate(ys) del X, Y Xte, Yte = load_CIFAR_batch(os.path.join(ROOT, 'test_batch')) return Xtr, Ytr, Xte, Yte def get_CIFAR10_data(num_training=5000, num_validation=500, num_test=500): cifar10_dir = r'D:\daima\cifar-10-python\cifar-10-batches-py' X_train, y_train, X_test, y_test = load_CIFAR10(cifar10_dir) print(X_train.shape) mask = range(num_training, num_training + num_validation) X_val = X_train[mask] y_val = y_train[mask] mask = range(num_training) X_train = X_train[mask] y_train = y_train[mask] mask = range(num_test) X_test = X_test[mask] y_test = y_test[mask] mean_image = np.mean(X_train, axis=0) X_train -= mean_image X_val -= mean_image X_test -= mean_image X_train = X_train.transpose(0, 3, 1, 2).copy() X_val = X_val.transpose(0, 3, 1, 2).copy() X_test = X_test.transpose(0, 3, 1, 2).copy() return { 'X_train': X_train, 'y_train': y_train, 'X_val': X_val, 'y_val': y_val, 'X_test': X_test, 'y_test': y_test, } def load_models(models_dir): models = {} for model_file in os.listdir(models_dir): with open(os.path.join(models_dir, model_file), 'rb') as f: try: models[model_file] = pickle.load(f)['model'] except pickle.UnpicklingError: continue return models这是一个加载cifar10数据集的函数,如何修改使其能加载mnist数据集,不使用TensorFlow
可以使用以下代码加载MNIST数据集:
```
import gzip
import os
import pickle
import numpy as np
def load_mnist(path, kind='train'):
labels_path = os.path.join(path, '%s-labels-idx1-ubyte.gz' % kind)
images_path = os.path.join(path, '%s-images-idx3-ubyte.gz' % kind)
with gzip.open(labels_path, 'rb') as lbpath:
labels = np.frombuffer(lbpath.read(), dtype=np.uint8, offset=8)
with gzip.open(images_path, 'rb') as imgpath:
images = np.frombuffer(imgpath.read(), dtype=np.uint8, offset=16).reshape(len(labels), 784)
return images, labels
def get_mnist_data(num_training=5000, num_validation=500, num_test=500):
mnist_dir = r'D:\daima\mnist' # 修改为mnist数据集所在的目录
X_train, y_train = load_mnist(mnist_dir, kind='train')
X_test, y_test = load_mnist(mnist_dir, kind='t10k')
print(X_train.shape)
mask = range(num_training, num_training + num_validation)
X_val = X_train[mask]
y_val = y_train[mask]
mask = range(num_training)
X_train = X_train[mask]
y_train = y_train[mask]
mask = range(num_test)
X_test = X_test[mask]
y_test = y_test[mask]
X_train = X_train.astype('float32') / 255
X_val = X_val.astype('float32') / 255
X_test = X_test.astype('float32') / 255
return {
'X_train': X_train,
'y_train': y_train,
'X_val': X_val,
'y_val': y_val,
'X_test': X_test,
'y_test': y_test,
}
```
这个函数将会返回训练集、验证集和测试集的图像和标签。其中,图像是一个形如`(num_samples, 784)`的数组,标签是一个形如`(num_samples,)`的数组。
def get_CIFAR10_data(num_training=5000, num_validation=500, num_test=500): cifar10_dir = r'D:\daima\cifar-10-python\cifar-10-batches-py' X_train, y_train, X_test, y_test = load_CIFAR10(cifar10_dir) print(X_train.shape) mask = range(num_training, num_training + num_validation) X_val = X_train[mask] y_val = y_train[mask] mask = range(num_training) X_train = X_train[mask] y_train = y_train[mask] mask = range(num_test) X_test = X_test[mask] y_test = y_test[mask] mean_image = np.mean(X_train, axis=0) X_train -= mean_image X_val -= mean_image X_test -= mean_image X_train = X_train.transpose(0, 3, 1, 2).copy() X_val = X_val.transpose(0, 3, 1, 2).copy() X_test = X_test.transpose(0, 3, 1, 2).copy() return { 'X_train': X_train, 'y_train': y_train, 'X_val': X_val, 'y_val': y_val, 'X_test': X_test, 'y_test': y_test, }这是一个加载cifar10数据集的函数,如何修改使其能加载mnist数据集,不使用使用 TensorFlow
要加载MNIST数据集,可以使用以下代码:
```
import numpy as np
import gzip
def load_mnist(path, kind='train'):
"""Load MNIST data from `path`"""
labels_path = f'{path}/{kind}-labels-idx1-ubyte.gz'
images_path = f'{path}/{kind}-images-idx3-ubyte.gz'
with gzip.open(labels_path, 'rb') as lbpath:
labels = np.frombuffer(lbpath.read(), dtype=np.uint8, offset=8)
with gzip.open(images_path, 'rb') as imgpath:
images = np.frombuffer(imgpath.read(), dtype=np.uint8, offset=16).reshape(len(labels), 784)
return images, labels
def get_MNIST_data(num_training=50000, num_validation=10000, num_test=10000):
mnist_dir = r'path/to/MNIST'
X_train, y_train = load_mnist(mnist_dir, kind='train')
X_test, y_test = load_mnist(mnist_dir, kind='t10k')
mask = range(num_training, num_training + num_validation)
X_val = X_train[mask]
y_val = y_train[mask]
mask = range(num_training)
X_train = X_train[mask]
y_train = y_train[mask]
mask = range(num_test)
X_test = X_test[mask]
y_test = y_test[mask]
# normalize images
mean_image = np.mean(X_train, axis=0)
X_train -= mean_image
X_val -= mean_image
X_test -= mean_image
# reshape images to 28x28x1
X_train = X_train.reshape(-1, 1, 28, 28)
X_val = X_val.reshape(-1, 1, 28, 28)
X_test = X_test.reshape(-1, 1, 28, 28)
return {
'X_train': X_train, 'y_train': y_train,
'X_val': X_val, 'y_val': y_val,
'X_test': X_test, 'y_test': y_test
}
```
其中 `load_mnist` 函数会从指定路径加载MNIST数据集,返回的 `images` 是一个形状为 `(num_samples, 784)` 的numpy数组,`labels`是一个形状为 `(num_samples,)` 的numpy数组。 `get_MNIST_data` 函数会调用 `load_mnist` 函数来加载数据集,并进行预处理,最后返回一个字典,包含训练集、验证集和测试集的图像和标签。
相关推荐
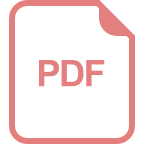
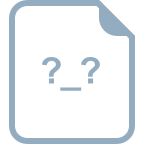












