python语言:使用pollard rho算法实现因式分解
时间: 2023-08-15 07:08:41 浏览: 77
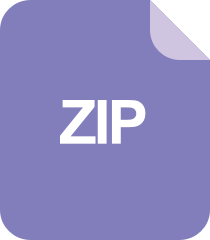
Pollard:Pollard 分解算法的基本python3 实现
好的,以下是使用 Python 语言实现 Pollard Rho 算法进行因式分解的代码:
```python
import random
def gcd(a, b):
while b != 0:
a, b = b, a % b
return a
def pollard_rho(n):
x = random.randint(1, n-1)
y = x
c = random.randint(1, n-1)
d = 1
while d == 1:
x = (x ** 2 + c) % n
y = (y ** 2 + c) % n
y = (y ** 2 + c) % n
d = gcd(abs(x - y), n)
if d == n:
return pollard_rho(n)
return d
def factorize(n):
factors = []
while n > 1:
factor = pollard_rho(n)
factors.append(factor)
n //= factor
return factors
n = 1234567890
factors = factorize(n)
print(factors)
```
在这个代码中,我们使用了 Pollard Rho 算法进行因式分解。首先,我们定义了一个辗转相除法的函数 gcd(a, b),用于求两个数的最大公约数。然后,我们定义了一个 pollard_rho(n) 函数,该函数接受一个正整数 n 作为参数,并返回 n 的一个因子。该函数通过随机选择 x 和 c,然后使用 f(x) = x^2 + c 的迭代公式来产生一个序列 x_0, x_1, x_2, ...,并使用 Floyd 算法来寻找序列中的循环节,最后找到两个序列中的相同元素并计算它们的差的绝对值和 n 的最大公约数,如果这个最大公约数不是 1 或 n,则表示我们已经找到了 n 的一个因子。如果这个最大公约数是 1 或 n,则需要重新选取 x 和 c,进行迭代。最后,我们定义了一个 factorize(n) 函数,该函数接受一个正整数 n 作为参数,并返回 n 的所有因子,该函数通过重复调用 pollard_rho(n) 函数来实现。最后,我们测试了这个函数,并输出了 n 的所有因子。
希望这个代码对你有所帮助!
阅读全文
相关推荐
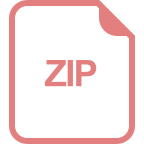
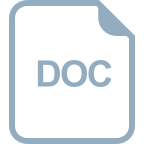














